Show Posts
|
Pages: [1] 2 3 »
|
#include <cstdint> #include <iostream> #include <cuda_runtime.h> #include <iomanip> #include <openssl/ripemd.h> #include <openssl/sha.h>
#define BLOCK_SIZE 1024
__global__ void hash160(char *pattern, unsigned char *out, uint32_t *nonce) { uint32_t index = blockIdx.x * blockDim.x + threadIdx.x;
unsigned char hash[SHA256_DIGEST_LENGTH]; char str[64];
for (int i = 0; i < 9; i++) { str[i] = pattern[i]; }
for (uint32_t i = *nonce; i < UINT32_MAX; i += gridDim.x * blockDim.x) { sprintf(&str[9], "%08x", i); SHA256((const unsigned char*)str, strlen(str), hash); RIPEMD160(hash, SHA256_DIGEST_LENGTH, &out[index * 20]); if (out[index * 20] == 0x00 && out[index * 20 + 1] == 0x00) { // Found a match! *nonce = i; break; } } }
int main() { char pattern[10] = "1abcdefg"; // 9 characters pattern
// Allocate memory on the host unsigned char *out = new unsigned char[3 * 20]; uint32_t *nonce = new uint32_t; *nonce = 0;
// Allocate memory on the device char *dev_pattern; unsigned char *dev_out; uint32_t *dev_nonce;
cudaMalloc((void**)&dev_pattern, 10); cudaMalloc((void**)&dev_out, 3 * 20); cudaMalloc((void**)&dev_nonce, sizeof(uint32_t));
// Copy input data to the device cudaMemcpy(dev_pattern, pattern, 10, cudaMemcpyHostToDevice); cudaMemcpy(dev_out, out, 3 * 20, cudaMemcpyHostToDevice); cudaMemcpy(dev_nonce, nonce, sizeof(uint32_t), cudaMemcpyHostToDevice);
// Launch the kernel hash160 << < 3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce);
// Copy output data from the device cudaMemcpy(out, dev_out, 3 * 20, cudaMemcpyDeviceToHost); cudaMemcpy(nonce, dev_nonce, sizeof(uint32_t), cudaMemcpyDeviceToHost);
// Print the results for (int i = 0; i < 3; i++) { std::cout << "Address " << i + 1 << ": " << std::setbase(16); for (int j = 0; j < 20; j++) { std::cout << std::setw(2) << std::setfill('0') << (int)out[i * 20 + j]; } std::cout << std::endl; } std::cout << "Nonce: " << *nonce << std::endl;
// Free memory delete[] out; delete nonce; cudaFree(dev_pattern); cudaFree(dev_out); cudaFree(dev_nonce);
return 0; }
blockIdx.x * blockDim.x + threadIdx.x undefinited why  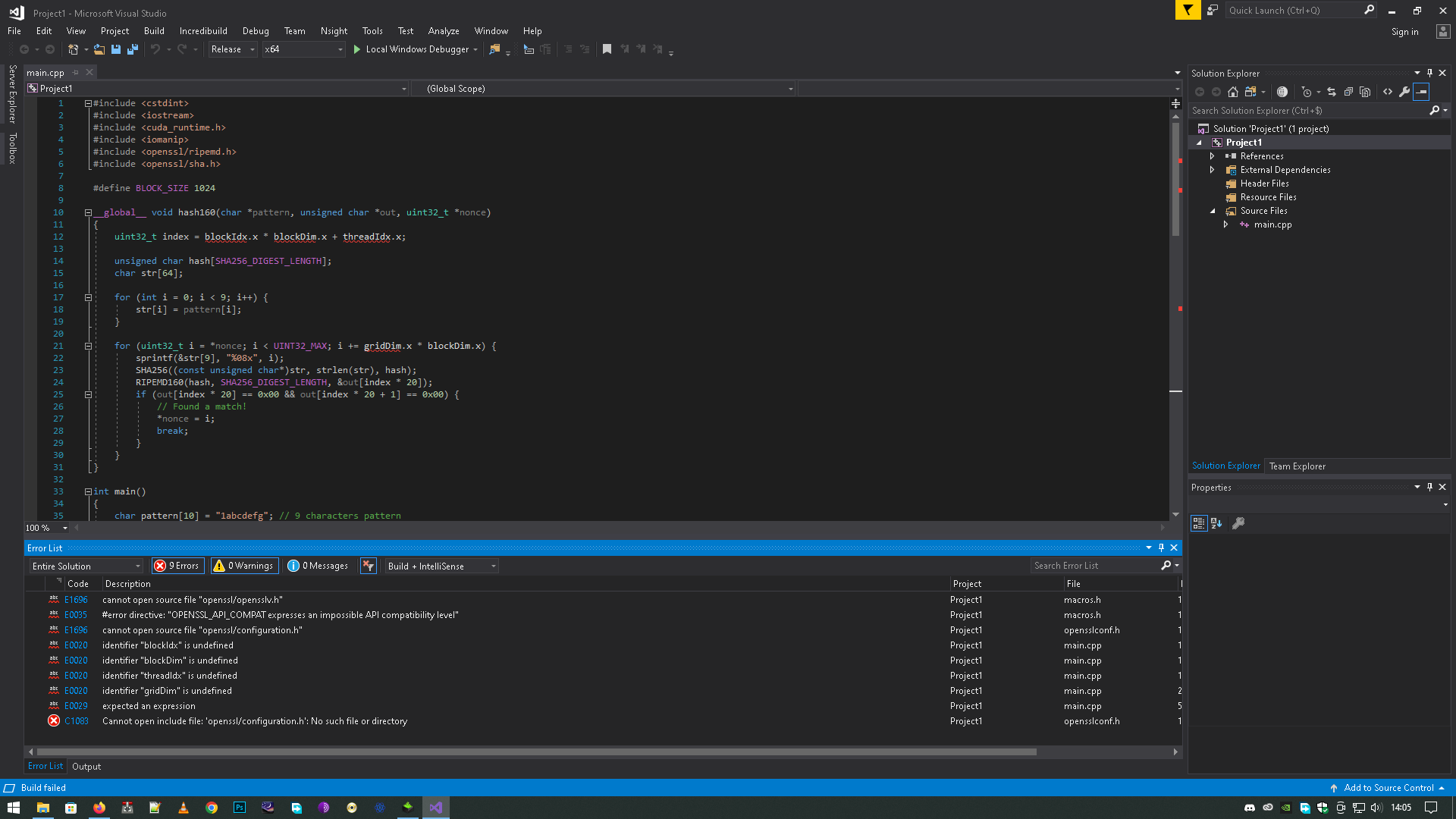
|
|
|
liste = [0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15]
while x == 97:
how many operation "+" can we have to obtain 97 knowing that only 17 numbers are allowed.
start with 3 + 5 + 15 numbers
|
|
|
hi from ecdsa import SigningKey, SECP256k1 from random import shuffle import datetime import time import mysql.connector import sys, os from binascii import unhexlify import hashlib from base58 import b58encode
t0 = datetime.datetime.now()
script_dir = os.path.dirname( __file__ ) mymodule_dir = os.path.join( script_dir, '..' ) sys.path.append( mymodule_dir )
def CompressedPublicKeyComputation(x, y): if int(y, 16) % 2 == 0: publicKey = '02' + str(x) else: publicKey = '03' + str(x)
return publicKey
with open("Luck.txt", "a") as f: print(t0, file=f)
def BitcoinClassicAddressComputation(publicKey): public_key_bytes = unhexlify(publicKey)
sha256 = hashlib.sha256() sha256.update(public_key_bytes) hash_temp = sha256.digest()
ripemd160 = hashlib.new('Ripemd160') ripemd160.update(hash_temp) hash2_temp = ripemd160.hexdigest()
hash3_temp = '00' + hash2_temp
checksum = checksum_computation(hash3_temp)
hash_final = hash3_temp + str(checksum) hash_final_bytes = unhexlify(hash_final) address = b58encode(hash_final_bytes).decode("utf-8") return address
def checksum_computation(string: str) -> hex: cs = hashlib.sha256(hashlib.sha256(unhexlify(string)).digest()).hexdigest() checksum = cs[:8] return checksum mydb = mysql.connector.connect( host="localhost", user="root", password="", database="bitcoin" )
g = 0 priv = list(range(1,10000)) shuffle(priv)
while priv: private1 = priv.pop() k = int(str(private1), 16).to_bytes(32, "big") k = SigningKey.from_string(k, curve=SECP256k1) K = k.get_verifying_key().to_string() h = (str(g) + ')\t' + str(private1) + '\t' + K.hex()[0:64] + '\t' + K.hex()[64:128] + '\n') chunks = h.split('\t') public_key = CompressedPublicKeyComputation(chunks[2], chunks[3]) public_key = public_key.split('\n') private_key = chunks[1] pub = BitcoinClassicAddressComputation(public_key[0]) print(private_key,pub) mycursor = mydb.cursor()
sql = "SELECT * FROM btc WHERE address='" + pub + "';"
mycursor.execute(sql) myresult = mycursor.fetchone()
if myresult: body = 'Private key= {pkwif} \r\n Address = {address} \r\n -----------------------' Message = body.format(pkwif=private_key, address=pub) fichier = open("Luck.txt", "a") fichier.write(private_key + ' ' + pub + '\n') result : 6108 1Ls1B3xCBFPRogcCVNSd9UT5LnorLutXhf 6108 is secret exponent but I would like my results in hex priv = list(range(1,10000)) to hex not possible
|
|
|
hi here my code : import time import random
elements = []
def fib(n): while len(elements) < 10000: priv = random.randint(10000, 20000) if priv not in elements: elements.append(priv) start = time.time() fib(1) end = time.time() elapsed = end - start
print(f'Temps d\'exécution : {elapsed:.2}s') result : Is it possible to do this while keeping the same execution time ? import time import random
elements = [] elements2 = []
def fib(n): while len(elements) < 10000: priv = random.randint(10000, 20000) priv2 = random.randint(20000, 30000) if priv not in elements: elements.append(priv) if priv2 not in elements2: elements.append(priv2)
start = time.time() fib(1) end = time.time() elapsed = end - start
print(f'Temps d\'exécution : {elapsed:.2}s') yet elements have 10000 entries as at the beginning
|
|
|
hi possible to generate a number by deleting what has already been generated in python without list try : import random
elements = str(random.randint(1, 100))
i = 0 while i != 100: selection = elements print(elements) print(selection) i = i + 1 elements.remove(selection) result File "1.py", line 12, in <module> elements.remove(selection) AttributeError: 'str' object has no attribute 'remove'
|
|
|
Hello, is it possible to generate bitcoin addresses very very quickly in this form?
PRIVKEY X Y 0x80687e340c49f738b5db81d3dc6f8b e84402e4b5677ca6a833073abb5ff75d5a5905a048f6dcb075c97230e81c8b0a c6f37535f8acf7e8ae56006c851ed6f94f4ff30d3f4df28f56af871a48f8600f
|
|
|
Could someone enlighten me on this subject because I can't figure out how these 18051648 addresses are generated. Thank you.
Subgroup Detection Since private keys are elements of Zq∗ , a straight brute force attack to the Bitcoin system seems infeasible, as inverting the map ϕ:Zq∗⟶,k⟶k⋅P would imply solving an ECDLP instance. However, there are few small subgroups H≤Zq∗ that may be inspected, for which an exhaustive computation of all the possible keys and corresponding addresses may be carried out. This way one may compute the inverse of the restricted map ϕ|H:⟶G. Since the keys are supposed to be uniformly distributed, there is no probabilistic argument suggesting their presence in specific small subgroups. However, assuming that this is the case, we need to choose a suitable subgroup. In this view, by considering the factorization of q−1 into prime integers q−1=26×3×149×631×107361793816595537p1×174723607534414371449p2×341948486974166000522343609283189p3,, it is not difficult to test that the maximal subgroup of moderate size (i.e. that can today be checked with an average computer) contains N elements, where N=26×3×149×631=18051648. Such a group may be easily produced by considering any primitive element t of Zq , such as t=7, and considering the element g=tp1×p2×p3, which generates the subgroup H=<g>={gi∣∣1≤i≤18051648}. Indeed, we summarize in the following theorem two well-known results. Theorem 1. Let F be a field. Then, any finite subgroup G≤F∗ is cyclic. Moreover, for every positive integer M dividing |G|, there is a unique subgroup H≤G such that |H|=M . Subgroup Inspection The group H as previously defined has less than 20 millions elements; therefore, we were able to straightforwardly construct, in a few days, the BTC addresses originated by all private keys k∈H and to check whether they have appeared in the BTC blockchain since its creation until 2018. We recall that an address appears in the blockchain whenever it receives any amount of bitcoin. Note that the number of addresses in the BTC blockchain does not correspond to the number of actual BTC users, as modern wallets handle many different addresses for each user. With this procedure, we found 4 BTC addresses, in which private keys belong to H:
1PSRcasBNEwPC2TWUB68wvQZHwXy4yqPQ3, 1B5USZh6fc2hvw2yW9YaVF75sJLcLQ4wCt, 1EHNa6Q4Jz2uvNExL497mE43ikXhwF6kZm, 1JPbzbsAx1HyaDQoLMapWGoqf9pD5uha5m.
Two of them, (3) and (4), came from the trivial keys 1 and −1 , and they might have been generated on purpose, but the remaining two addresses appear to be legit. In particular, a blockchain inspection (Reference [21], 2018) suggests that one of them (2) has been used as temporary address for moving a small amount of bitcoins, while the other (1) has probably been used as a personal address, since its owner has stored some bitcoins there for 4 years. To show that the private key of address (1) was really recovered, we used three of our addresses
A. 1FCuka8PYyfMULbZ7fWu5GWVYiU88KAU9W, B. 1NChjA8s5cwPgjWZjD9uu12A5sNfoRHhbA, C. 1695755gMv3fJxYVCDitMGaxGu7naSXYmv,
and we performed tiny transactions from each of them, as shown in Figure 3. These operations may be easily verified through any blockchain explorer, such as Reference [21], by searching for their transaction IDs:
T1. 69ad7033376cea2bbea01e7ef76cc8d7bc028325e9179b2231ca1076468c1a1e, T2. 1dd5c256a1acc81ea4808a405fd83586ea03d8b58e29a081ebf3d0d95e77bf63, T3. b722c77dcdd13c3616bf0c4437f2eb63d96346f74f4eeb7a1e24c1a9711fc101.
|
|
|
Hello Lately, I have created an absolutely sublime crypto casino. Small demo available right here: https://demoscript.online/ 18 games available, a crispy admin panel and hundreds of options like the biggest online casinos. The source is custom ~50% to have something nice and clean and unique. It is made in PHP with Laravel and for the db it is MongoDB. I will set a quota of 0.01 BTC which seems reasonable to avoid the leeching of such a beautiful project.
|
|
|
Hello, I would like to know how to decipher this
and what values would change for the next block ?
01000000010000000000000000000000000000000000000000000000000000000000000000fffff fff0704ffff001d0104ffffffff0100f2052a0100000043410496b538e853519c726a2c91e61ec1 1600ae1390813a627c66fb8be7947be63c52da7589379515d4e0a604f8141781e62294721166bf6 21e73a82cbf2342c858eeac00000000
01 version ect......
thanks
|
|
|
Good evening everyone first of all I would like to know with ecctools, the maximum division is how much?
I take an example
there are 150 public keys so the maximum division will be 150
|
|
|
Good morning,
I would like to know if it is possible to redesign the bitcoins addresses like this:
base58 = 123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxyz
creation of the private key base58(privkey)
base57 = 123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxy 'z' removed
base57(address(privkey))
1st) is this possible? 2nd) if the generated private key gives a bitcoin address that must contain a 'z' how will this be interpreted? 3rd) for a generated private key would it be possible to scan only the bitcoin addresses that do not contain the 'z'?
|
|
|
Hello, I would like to have more information about the public key:
By choosing half of 2^256 private keys:
7fffffffffffffffffffffffffffff5d576e7357a4501ddfe92f46681b20a0 has for public key: 03000000000000000000000000003b78ce563f89a0ed9414f5aa28ad0d96d6795f9c63
the public key is a little strange but let us say that it is simply the symmetry which makes that and thus if I increase of 1 the private key: 7fffffffffffffffffffffffff5d576e7357a4501ddfe92f46681b20a1 it has for public key: 0300000000000000000000003b78ce563f89a0ed9414f5aa28ad0d96d6795f9c63
what is normal is the symmetry.
the strange thing is that if I add 1 to the public key : 0300000000000000000000003b78ce563f89a0ed9414f5aa28ad0d96d6795f9c64
apparently it exists because it is connected to this bitcoin address: 13vSJ5pXUcUqiKoFN1gm7ffHVpK45G7b3e.
My question is this: Does this public key really exist or not ? And would they be positioned at strategic points ?
because if I continue it works
0200000000000000000000003b78ce563f89a0ed9414f5aa28ad0d96d6795f9c68 1LQiRWwELhChkpuAaC6tFpSDqpdjR3fYiC 0200000000000000000000003b78ce563f89a0ed9414f5aa28ad0d96d6795f9c6a 172N3FM1uEjzSx28Tg1aoSzDqgmd9yqqCa ...........
|
|
|
Does anyone know the public key mirror ?
|
|
|
hi would anyone know how to get all the bitcoin outputs listed ?
|
|
|
Hello I would like to know if from a private key we could know all the addresses of type "1" "3" "bc" ....... if yes how?
|
|
|
Hello we know that there are 2^96 identical bitcoin addresses in the 2^256 private key. Is it the same for the public keys?
|
|
|
|