Hello mining friends

I would like to tell you about my experiences, adventures and impressions I have made in the last weeks/months
regarding solo mining. This should serve as a guide but also as a review for like-minded people who are facing similar problems and are looking for answers or want to share their own experiences. It is not meant to be a manual, as it would go beyond the scope, although I will elaborate on some points I consider worth mentioning. If you want to learn more about the technologies presented here, you are of course free to do your own research and, if you have any questions feel free to create new posts of your own about them. For the following thread I would like to
emphasize that all impressions I describe in the course are purely
subjective, they show
my personal opinion and this does not imply that it has to correspond to the fact or other views. I also refer exclusively and without exception always to Bitcoin, as I do not care about alt-/shitcoins and thus do not support them.
1) Requirements --> hash power for SHA-256 
.
An indispensable condition for solo mining is -who would have thought it- hash power! Either you own your own hardware like ASIC miners (e.g. Bitmain Antminer, MicroBT Whatsminer, Canaan Avalonminer, etc.)
or USB miners specially developed for the SHA256 algorithm (e.g. Gekkoscience Compac F, NewPac, etc.)
or you
rent the required hash power from one of the two well-known providers/marketplaces:
MiningRigRentals.com or
www.NiceHash.com. The principle behind these hashpower marketplaces is simple: ASIC miner owners can choose to use their hashpower themselves for mining or instead offer all or part of their device's hashpower for rent from MRR or NH. Understandably, the rental prices on the marketplaces are slightly higher than the rewards the ASIC miner owner would receive if they were to mine with PPLNS/PPS themselves. Therefore, many ASIC miner owners offer their hashpower for rent. Customers, on the other hand, are happy to accept the extra charge because they can scale very precisely when and how much hashpower they need. In addition, everything is included in the agreed rental price, the renter does not pay for electricity and does not have to deal with the loud noises that such an ASIC miner generates during operation.
Since I don't own any mining hardware at this point, I have to rent the hash power I want. I couldn't decide at first, but after reading a lot of forum posts here, or on Reddit and other sources, I initially chose NiceHash, simply because there was more reading material and experience reports. It gave me the impression that NiceHash was bigger/well known.
2) NiceHash.com (abbreviated as NH in the following)So first I created an account with NH and tried to get an overview. First I "funded" my account with a not insignificant
BTC amount by transferring from my private wallet to the NH wallet of my account. Nowhere in the deposit process does NH mention that 2FA is mandatory for a later withdrawal, but more on that later. Then I wanted to rent Hashpower from NH. Unfortunately, this turned out to be anything but easy, as I find the system rather confusing and not intuitively self-explanatory. The list presented under "Hashpower Marketplace" is difficult to interpret for a newcomer at first glance. There are roughly structured two markets displayed called "USA - WEST" and "EU WEST". For each of these 5 columns are shown: <Order ID>, <Price in BTH/PH/DAY>, <Limit in PH/s>, <Miners> and <Speed>, see the following screenshot.

For a newbie not everything is understandable and certainly not intuitive, because you can't click or even select anything at first glance. If you look at this line, for example ...
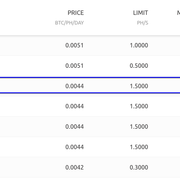
Then you understand that the ORDER ID is an 8-digit hex value that is supposed to hide the origin of the provider(s) for anonymity reasons. The column "PRICE per BTC/PH/DAY" is also understandable, it simply means that per Petahash 0.0044 BTC will be charged for the rental period of 24h. What the column "LIMIT" and "MINERS" should express, however, was mysterious to me at first. Afterwards I could find an explanation in the
NH help pages. There it is explained that with NH you do not explicitly rent a single ASIC miner from a single person X, but that it is hashpower put together from several providers and machines. With
Limit, it sort of sets the upper limit of hashpower in PH/s that one can choose when booking. And
Miners shows how many individual mining machines this offer consists of.
After a bit of trial-and-error, I found that if you hover over the "Price BTC/PH/Day" column, a green checkmark with an arrow appears, and if you touch it with your mouse pointer, the text "Outbid order" appears. And here comes a
crucial information to keep in mind when using NH:.
In the NH marketplace, everything is based on the system of bid and ask, i.e.
the highest bidder gets the hash power. So you have to place your bid to rent the hashpower of a rig. So if one has placed an order and received hashpower and it has been sent to the desired pool, then everything is going so far for now. However, if you are suddenly outbid
by someone else on the NH marketplace, then you have no hashpower anymore and are left standing because the highest bidder has "
snatched" this hash power away. He simply bid more for it, so he got the hashing power. Of course, this is then no longer charged for and you also only pay for the service you receive, at least that's how fair it is. As soon as the other higher bidder is done or is no longer willing to pay as much and quits and you are therefore at the top of the NH queue, it continues again and you receive hash power again. Then the billing also continues accordingly. Despite everything, this condition should always be taken into account for one's own planning.
My overall impression and conclusion about Nicehash:for
several reasons for me an
absolute no-no:
-
in order to be able to rent hashpower from NH, the customer is forced to go through KYC and drop his pants. Since I do not support this in principle
and am strictly against it, it was already the KO criterion to continue to operate on this platform. I must honestly confess that I should have informed myself more thoroughly on their help pages beforehand. But I didn't even begin to expect that "renting hashpower" would require KYC for such insignificant simple actions. I was obviously mistaken.
- i wanted to withdraw the high
BTC amount back from NH by transferring it back to my private wallet. However, that was
not possible and caused further trouble.
NH forces one to set up two-factor authentication (2FA) to transfer amounts out of NH anywhere Bottomless cheek! Funnily enough, I was
not forced[/i] to 2FA when I made the
deposit, they accepted that without a hitch. But when paying out, they deliberately make it difficult. In short: To deposit funds into the NH platform they like to see, to release amounts that leave the NH platform again --> they obviously don't like to see that and make it more difficult for the customer intentionally by such hurdles. I don't like such "policies", that is for me
no-go!.
- I have followed dozens of solo mining parties that were carried out using hashpower on Nicehash. In doing so, I myself was able to follow the progress of hash power, shares, best share, etc... in detail, even though you don't have to have participated yourself. More about this later, in the section Kano-Pool. A run of about one day shows dozens of dropouts, interruptions and partial standstill for several hours. The reason for this was already mentioned above ->
NH works on a bidding system and if someone overbids then the hash power is "snatched" away from you and you are left with 0 hashes/second. This is
unreliable for the following reasons:
- (a) Let's assume a big difficulty increase of the bitcoin network is coming up in the next 25h, which everyone can see and estimate. But I still want to try my luck in the current difficulty class and mine for 24h with a huge hashing power. I rent this with NH and off I go. After about 6h I get outbid and so I don't hash at all. The one who outbid me is done after 17h and I slip back to the highest bidder and get my booked computing capacity again. This would theoretically run for me for another 18h (6h I have already used) but at this point after 1h the difficulty has been increased and I have no more interest in mining due to the blatant difficulty increase. I stand there stupidly, bag to, thing run. Finally, it means for me as a renter, I can not set a guaranteed rental period and thus can not reliably plan and calculate in the long term.
- (b) I can't do proper costing and long-term planning because the potential rental of hashpower has no 'fixed' costs can be allocated. I don't mean the general and usual market rice fluctuations but simply the fact that I don't have stable values for calculating rig/hashpower rent due to this supply/demand system. This is the logical conclusion from point a) because both price and time are assigned as a function.
- (c) There is no evidence or criteria in NH that meaningfully represents the state of a rig (or rig aggregation that is behind the anonymized "Order ID") to enable an assessment of reliability. Due to the lack of a rating system, it is not possible for the customer to roughly estimate whether the rigs will actually work reliably for the rented period without considerable hashrate fluctuations or even failures when they go offline (for whatever reason). In my opinion there is only one small approach to counteract this -> The value of the column "Miners" from the offer list should be as high as possible when selecting an Order ID. This way it does not matter much if e.g. 2 out of 139 miners would fail.
3) MiningRigRentals (hereafter abbreviated to MRR)Since it was crystal clear to me that I would never use Nicehash for the first time, that left only MRR, easy choice

So, registered there and looked around what MRR has to offer. Right away I got along much better here, everything worked intuitively and it was very clear to my sensation. Click on [Marketplace] and then click in the search box and start typing, when you get to SHA2.... all three available algorithms are displayed as hits:
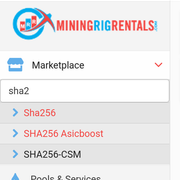
Relevant to Bitcoin, the two hits colored in red are "Sha256" and "SHA256 Asicboost", with the latter offering better rents and usually being cheaper.
Here is a sample overview for the SHA256-ASIC rigs (as of 10/15/2022 / around 7:30pm):

When you select a rig, you get quite a bit of information displayed about it and can get an idea of whether it is a reliable rig and will keep the offered hash rates over the long term. For example the following rig (freely chosen by me, is now not necessarily a favorite of mine, so just random selection for demonstration purposes):
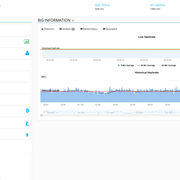
We see the location (US-EAST) for this rig. This is not irrelevant, as some pool operators allow you to choose certain pool servers located in other regions / countries / continents. The goal should be to have the shortest possible latency between the rig and the pool, as this can be the deciding factor for (failure) success. If I rent a rig in Russia and send the hashpower to a pool on the US west coast, it could be that the connection is much worse than choosing a pool server in the EU. In the worst case it can be that you have found a block, but until it is fed into the network and propagated it took too long, someone else was faster and you stand there stupidly without reward (orphan block). I just want to point out that it can be helpful to know the server location of the rig so that you can later adapt it to the server locations of the mining pool you are using, if the pool you are using offers this possibility at all. In the screenshot we also see the operator of this rig, I find this very helpful, why? I maintain an excel list for all my rentals I have done so far. I can make notes on them and very quickly and accurately filter out the good rigs from the bad. If I see certain "owners" with whom I have had bad experiences in the past because they responded late or even unfriendly to my inquiry about a problem, then I set internally "red flag" on these owners or also specifically on the Rig ID. I then avoid renting the same owner or rig again in the future. In the field "Suggested Diff" the rig owner can enter a Difficulty, which should be used as a guideline. So the person who wants to rent the rig knows if it is a lowDiff or HighDiff. In some pools you can also set the Diff, so you can select the right adjustment in advance so that everything goes smoothly in highspeed. The value under "Optimal Difficulty" is automatically calculated by MRR for the respective rig in such a way that 2-3 shares per minute are processed. This ensures the best possible throughput and a solid average performance. If the difficulty would be tuned higher, less shares per minute would come through and this would cause a rather fidgety course of the plot in the long run (also in the charts). The charts are an important basis to show reliability. In the above posted screenshot you can see in the upper chart window the live hashrate for the short-term time range.The blue area shows the 5min average and thus the largest swings. The black line the 30min average and the green line the 60min average. For the long-term view and thus the reliability one looks at the lower chart. The blue filled area shows the short term 5m average at the time shown, the black line the 30m average with a more smoothed line. Also, the red dashed line in both chart areas marks the hashrate advertised by this rig (500 TH/s in the example). So you look at the bottom chart, the black line should be at least above or around the red dashed line. This gives you great reliability that the advertised hashrate will be consistently achieved and delivered over the long term.
The upper chart shows the live hashrate, it serves as a quick assessment of whether everything is running ok or there could be any problems. In principle, it behaves like this: it may be that the rig briefly delivers less than the 500 TH/s advertised here, but it delivers a higher hashrate sometime later. Here again a screenshot I made a little later from the same rig. There you can see that the live hashrate was once briefly below the red-dashed one, but then shot up again and delivered 634 TH/s. The averages are relevant.
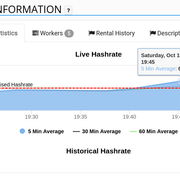
In the example, you have to rent for at least 24h and can rent for a maximum of 72h. The lowest possible rental period for MRR in general is 3h and on top there is no fixed limit as far as I know, or the rig operator can set it very high. Through the Quick Calculator you can see immediately how much BTC it would cost if you would rent xx hours and yy minutes. What is also possible: you can extend
during the rental period if you want to. That however to a small extra charge, which is accounted for in the form of a fee. However, this is displayed transparently when you want to book the "extension".
The tabs [Workers] and [Rental History] are also very useful. In "Workers" you can see directly which difficulty the respective worker is currently using and how the individual hash rates of the particular workers look like. This can be very useful if you use a pool that allows customDiff. You can see to what extent the rig and the pool have synchronized and work well or less well together. You can also see exactly which miner software/firmware and which ASIC miner is being used and also the location of each worker. In the [Rental History] tab you can get a good picture of how reliable the rental operations have been in the past. Green color means that the advertised hashrate was largely achieved (plus minus about 5% is within the tolerance range, I think). These values also form the RPI (Rig Performance Index), a benchmark whether the rig is reliable and delivers the advertised hashrate in the long run or not. You can also see how long the rigs have been rented in the past and also by which renter (unless the user has turned off this feature under their personal settings, so that
anonymous is then displayed there).
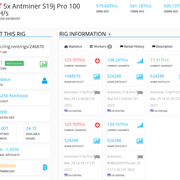
Now let's move on to the pools and how to choose one ...
4) Which solo mining pool to choose?I had initially looked at
<https://btc.com/stats/pool?pool_mode=all> to get an overview of all available pools. However, it lists all possible pools that have already mined blocks. You can't see if the listed pool also offers solomining. Nevertheless, the information on this page is interesting, more on this later.
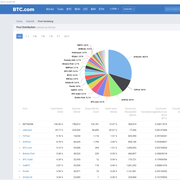
The pool that found the most blocks is "
Unknown". Unknown because it contains all those mined blocks whose coinbase transacation content could not be matched to any known pool string. For example: If user "lucky_miner" is mining privately on his own full-node and finds a block, then he can write free text into the coinbase transaction, which then appears publicly in the coinbase transaction of that mined block. However, the major known mining pools (F2Pool, Antpool, Slushpool, Binance, ViaBTC, SoloCK...) use specific text patterns for the coinbase transaction and therefore unique identification is possible. In short, all blocks mined that cannot be uniquely identified and assigned to a pool appear as "Unknown". The top of this pool list is "F2Pool" as can be seen. After that comes "AntPool", "BTC.com", "Braiins Pool" (formerly Slushpool), "BTCguild", "ViaBTC", "Poolin", etc. The actual total hash rate of the pools cannot be taken from this page, but is completely irrelevant for solo mining.
Originally I thought that the column "
Empty Blocks Count" or expressed in percentage "
Percentage of Empty Blocks", shows the number of blocks that the pool lost and therefore did not pay out. But empty blocks are just blocks without any transaction except their own coinbase transaction. So it is still a mystery to me why this "empty blocks" column matters and is displayed on this page at all. Another important thing are the "stale shares". These are shares that the worker returned to the pool too late. An "orphan block" instead is a completed and
actually valid block whose calculated hash value was below the difficulty target and thus would be valid,
but it was propagated too slowly through the network. Someone else promptly also found a valid hash value and thus a valid block, and propagated it faster than you. The longest chain wins, the inactive part that did not make it is ignored by the network and a way (orphan) is formed, the too slowly propagated block is thus dropped, hence "orphan". For the miner, of course, this is a nightmare, as he has rejoiced too early. Therefore, miners and especially beginners should always be aware that it is actually a bad idea to mine from home with your private internet connection (DSL, cable) and your full node, even if you should have 1 GBit/s fiber connection or cable connection. It is and remains a private household and the connections to the Internet are always inferior to a connection of a server in the data center. The private computer goes through dozens of routers (hops) and gateways and its bandwidth is shared with hundreds/thousands of other users in the strand. Both the ASIC miner and the full-node should therefore be located as close as possible to a performant backbone. In addition to this, the novice miner may unknowingly further aggravate his position by using VPN or TOR connections for his mining project, or even WiFi. All reputable mining pools invest in potent hardware with a damn fast internet connection in different and thus multiple data centers/locations, with DDoS protection and much more.
For now, let's move on to the next info page to help us choose a pool for solo-mining...
https://MiningPoolStats.stream/bitcoinThis page shows us all pools in the default view sorted by the total hashrate of the pool. The data is obtained through API from the respective pools. Some pools simply don't have an API or deliberately don't want to query data from such services (e.g. Foundrydigital). Here is an example screenshot of what this looks like:
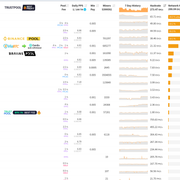
On top here is foundrydigital, as a private person I don't think you can participate there at all, here are really big fish on it with millions / billions of expensive equipment and really big mining farms. There are certain requirements here, so that one can participate in the pool at all. Therefore, there is no information about the different mining types that are provided, or returns, or number of miners. The other places in the top 5 are usually shared by Antpool, F2Pool, ViaBTC and Binance. So how do you use this information to choose a suitable pool for your mining project? Well, that depends on if you want to do solo mining or if you want to mine as PPS+ or PPLNS in a community. I had dedicated myself to all types and made my experiences with it.
However, this thread is supposed to be exclusively about solo mining, so I focus on filtering out the pools that offer solo mining.
We look in the [pool/fee] column and look for the pools that have the text
SOLO with red font color. Next to it is the fee that goes to the pool operator. The information "Miner Count, DailyPPS, Min.Pay, Hashrate, Blocks, LastFound" are
all irrelevant for solo mining.

I'm picking out the
solo mining pools:
5. ViaBTC.net (1 %)
21. zsolo.bid (0,5 %)
22. luckymonster.pro (0,5 %)
23. btcmill.cc (1 %)
24. solo.ckpool.org (2 %)
26. kano.is (0,5 %)
27. mining-dutch.nl (1,5 %)
30. solomining.io (1 %)
36. soloblocks.io (0,4 %)
38. aminingpool.com (0,95 %)
43. prohashing.com (1,99 %)
... and sort ascending by pool fee:
soloblocks.io (0,4 %)
zsolo.bid (0,5 %)
luckymonster.pro (0,5 %)
kano.is (0,5 %)
aminingpool.com (0,95 %)
solomining.io (1 %)
btcmill.cc (1 %)
ViaBTC.net (1 %)
mining-dutch.nl (1,5 %)
prohashing.com (1,99 %)
solo.ckpool.org (2 %)
Most would probably intuitively opt for those with the smallest fee, so that they have to pay as few fees as possible in the event of a block hit and reward receipt and thus more goes into their own pockets. But whether all that glitters is gold? What I describe now shows
my approach and thoughts to it. The whole thing should ultimately serve as a suggestion and idea. I rented a cheap rig from MRR for the short duration of 3h and a hashrate of 100 TH/s which I then directed to each pool in turn and took some meaningful screenshots and documented my experiences. I tested each solo pool from this list so that I could filter out and then ultimately settle on one.
How do I evaluate and what criteria matterWebsite:First impression of the website? Does it look similar to already known websites or pools then I devalue it because the operator probably wanted to save time and quickly copied a layout and changed it slightly. I know that there are self-titled "crypto experts" on the Internet who offer as a service a ready installed and configured mining pool for a fee (by abusing open-source pools for this, ugh). They offer different packages at different prices, depending on the functionalities. Such layouts are immediately noticeable, especially if the operator has made an effort for customizing or not. I downrate if the website requires JavaScript. I usually rate positive, if a clean and neat design stands out, so that everything remains clear. I like it plain and simple, without a lot of bells and whistles and dozens of buttons and tabs or submenus. So that even on the go with the mobile phone can be accessed directly and quickly. I find it positive when the statistics data of the own miner can be called directly via URL. I rate it positive if I get the impression that the pool operator has made an effort to list as much
helpful information as possible. I radically devalue if you are forced to
register before using the respective pool.
Server location and availability:.
Most pools offer at least 1 server and 2 different port numbers (see following paragraph "Difficulty/Configuration"). A few of them offer additional port numbers, e.g. 443 or 25, which can be advantageous for some constellations, depending on where the miner is located and where it might not be possible or even allowed to connect via the high ports (>1024). Port 25 (SMTP) and 443 (TLS) are allowed by routers/firewalls with higher security. It could be that you have your own miner somewhere on a company site, but the network there blocks all outgoing traffic on unknown ports. In such a case you could try using port 443, as this - with a bit of luck - would be treated less restrictively by the company firewall. Furthermore, MRR requires that you have at least two servers configured as a pool so that in case of a pool problem MRR interface can automatically switch to the other one. Only then you are entitled to a refund in case of problems and complaints. I consider it positive if alternative ports are offered by the pool. It is also a plus if the pool offers not only one server but several distributed over the continents, preferably all as high-end computers in data centers with direct backbone connection.
Difficulty Configuration:All pools I know of offer at least the "varDiff" functionality. This means that the pool gives the connected miner the jobs with an initial difficulty (initial diff) and then looks how long it takes. Most pools aim for about 2-3 shares per minute. They increase or decrease the difficulty accordingly, it's kind of
automatically negotiated and coordinated between the two parties. However, if the "initial diff" seems too large for the miner during the initial negotiation by the pool, the two do not get on the green branch. Miners with higher computational capacities can understandably handle higher difficulties and, above all, faster. They need a high diff. That's why all known mining pools offer their users the option to use either lowDiff or highDiff. This is achieved by connecting to a different port number. This is described in the respective instructions of the pool. Then there is additionally the functionality "custom diff", this gives the user the possibility to define and set the desired difficulty manually, mostly via the password field in the format "d=123456" where the abbreviation 'd' stands for difficulty and the value is the desired value you want to configure. However, this should only be necessary in special cases, the automatic tuning usually works quite well, as long as you have chosen lowDiff or highDiff correctly. "VarDiff" and "lowDiff/highDiff via port number" is actually part of the standard, customDiff is not offered by all. I therefore consider it a small plus.
Freely selectable worker names to distinguish the individual runs:.
Most, but not all, of the solo mining pools presented here allow users to use user-defined worker names. These worker names are appended as a suffix to the user's own Bitcoin payout address, using a period (.) or underscore (_) as a separator. Example: the own bitcoin address to be used as payout address is bc1q111222333444 and the desired worker name would simply be bc1q111222333444.foo or bc1q111222333444_bar etc. Why do this? Quite simply, to distinguish and clearly separate the statistical data collected for the particular run for further analysis. For example, I run a solo run today by renting the hashpower 500 TH/s for 6h and pointing it to the solo pool and then I see in the statistics data of the pool how many shares I managed and what my highest share (best diff share) was. Let's say my 'best diff' was 271G. Tomorrow I rent hashpower again and send 2 PH/s for 12h to the same pool with my same bitcoin address. Inasmuch as I would not have exceeded the 'best diff' value of yesterday's run (of all previous runs), I would never see what my 'best diff' was for the current twelve hour run at 2PH/s if it was less than 271G. That's because the statistics would still display 271G. Also I can't distinguish and read out how many shares were transferred in the current run. Simply because each time
the same bitcoin address was used and the data is cumulated (= merged). This circumstance can be circumvented by choosing worker names. In this case I would have chosen the worker name bc1q111222333444.500TH-6h for the first run and bc1q111222333444.2PH-12h for the second run to distinguish them... at least that's how
I do it, of course it's up to everyone how to choose and handle worker names
Direct mining via own Bitcoin address without registration:.
Absolute no-go is when the solo pool forces the user to register. It is not a matter of having to register with an e-mail address, but of mining using a user name that was used during registration. Consequently, in the event of a block discovery, the reward of currently 6.25 BTC is not paid directly to the user's own Bitcoin address, but only to the defined payout address specified by the solo pool owner. The pool operator has defined this statically for his pool, and this data is stored in the coinbase transaction. In practice, in case of a block hit this means that the pool operator collects the reward of 6.25 BTC + transcation fees in the first step itself and then only pays out to the respective user to the payout address requested by the user and entered in the customer portal. In the worst case, the mining pool operator could bitch and refuse the payout or put other hurdles in the way, such as forcing the user to first perform KYC before he pays out to him. Or the operator might die in an accident or due to illness, or the company (the solo pool) goes bust and everything ends up in a bankruptcy estate and you can only hope to get your reward that you are actually entitled to. I would definitely
not take such risks with such high amounts of currently > 6.25 BTC. All pools without exception that do not allow direct mining with one's own private Bitcoin address are NO-GO and
red flag, even though I included them for comparison in this test.
Statistics data and information on solo mining:.
While not a statement of potential success for mining a new block, I personally find the "best diff" and "best ever" stat info to be entertaining. "Best Diff" is the highest value achieved for one's bitcoin address/worker and is automatically reset to zero by the pool should the respective solo pool find a new block (regardless of which user on that solo pool). The "best ever" however remains, it will never be reset and shows the personal 'highscore' that has ever been reached under the given payout bitcoin address. You get a clue how good or bad your own run went and can compare in the future. Other useful information would be 'number of shares' and the 'current Difficulty' that the pool gives out to its workers. This is for performance purposes, you can see how many shares/min are processed at which diff. Accordingly, you could set the Miner<>Pool like this with e.g. customDiff to adjust these works to your taste and liking. Although it shows nothing about the chances of success, some may want to do it themselves and customize, so a lot of information provided is only an advantage and never a disadvantage. In the end, those who don't need certain info can simply ignore it. In short, if the pool provides as much info/statistic data as possible, then I consider that a plus.
Background information about the operator, presence and notoriety in the scene:.
I search the internet for all conceivable information about the pool in question. Primarily here on bitcointalk of course but I also look for clues or experiences on Youtube videos, Reddit posts, IRC servers or log onto their Discord servers and follow the conversations for several days/weeks.
Number of users+workers:
I look at how many users have joined this pool and tried their mining luck there. The pool operator could of course easily fake this data on their own pool website, but I still consider it as an approximate guideline to get an idea if the pool is in demand or if it's more of a flash in the pan. The overall hash rate of a solo pool has no significant meaning for a solo miner, as this performance has no relation to the success or failure for solo mining. It only says that a few or more miners are sending hash power to that solo pool to try their mining luck. Likewise, the indication of "users" or "workers", this only serves as a rough guide to assess the pool acceptance of the mining community.
Solo Pool Success StoryProbably the most critically considered and most important question in this context is: "Has the respective solo mining pool ever been successful, has a block already been found here and has the corresponding miner received his payout without complaint?" For this purpose, one can primarily make use of
this BTC pool list, the interpretation of which, however, requires explanation so as not to mistakenly compare apples with oranges. One must know here that with this list
all pools are listed without differentiating between solo pool mining or
community pool mining where people mine
as a group via PPLNS/PPS/etc. There are pools that offer
both models (e.g. ViaBTC or Kano Pool). If you look at place 29 "KanoPool" in this list, for example, there is a value of 2,433 blocks in the "mined blocks" column. However, this
does not (!) mean that so many blocks were mined in the KanoPool
via solo mining. In reality, the Kano.is pool as solo mining
has not found a single block so far. It has also only had the solo mining model in place about 1 year ago, prior to that it offered its services exclusively as PPLNS for years. The blocks shown were mined in the PPLNS model, the last block found in PPLNS mode was found by Kano on 10/25/2021 (
see details here). Likewise, this list also does not tell us how many blocks the large
ViaBTC pool found in solo mining mode. The data on this list is commingled,
therefore no meaningfulness of the "mined blocks" column in terms of solo mining, so this important info should be kept in mind.