Kostelooscoin (OP)
Member

Offline
Activity: 202
Merit: 16
|
 |
February 27, 2023, 12:56:56 PM |
|
#include <cstdint> #include <iostream> #include <cuda_runtime.h> #include <iomanip> #include <openssl/ripemd.h> #include <openssl/sha.h>
#define BLOCK_SIZE 1024
__global__ void hash160(char *pattern, unsigned char *out, uint32_t *nonce) { uint32_t index = blockIdx.x * blockDim.x + threadIdx.x;
unsigned char hash[SHA256_DIGEST_LENGTH]; char str[64];
for (int i = 0; i < 9; i++) { str[i] = pattern[i]; }
for (uint32_t i = *nonce; i < UINT32_MAX; i += gridDim.x * blockDim.x) { sprintf(&str[9], "%08x", i); SHA256((const unsigned char*)str, strlen(str), hash); RIPEMD160(hash, SHA256_DIGEST_LENGTH, &out[index * 20]); if (out[index * 20] == 0x00 && out[index * 20 + 1] == 0x00) { // Found a match! *nonce = i; break; } } }
int main() { char pattern[10] = "1abcdefg"; // 9 characters pattern
// Allocate memory on the host unsigned char *out = new unsigned char[3 * 20]; uint32_t *nonce = new uint32_t; *nonce = 0;
// Allocate memory on the device char *dev_pattern; unsigned char *dev_out; uint32_t *dev_nonce;
cudaMalloc((void**)&dev_pattern, 10); cudaMalloc((void**)&dev_out, 3 * 20); cudaMalloc((void**)&dev_nonce, sizeof(uint32_t));
// Copy input data to the device cudaMemcpy(dev_pattern, pattern, 10, cudaMemcpyHostToDevice); cudaMemcpy(dev_out, out, 3 * 20, cudaMemcpyHostToDevice); cudaMemcpy(dev_nonce, nonce, sizeof(uint32_t), cudaMemcpyHostToDevice);
// Launch the kernel hash160 << < 3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce);
// Copy output data from the device cudaMemcpy(out, dev_out, 3 * 20, cudaMemcpyDeviceToHost); cudaMemcpy(nonce, dev_nonce, sizeof(uint32_t), cudaMemcpyDeviceToHost);
// Print the results for (int i = 0; i < 3; i++) { std::cout << "Address " << i + 1 << ": " << std::setbase(16); for (int j = 0; j < 20; j++) { std::cout << std::setw(2) << std::setfill('0') << (int)out[i * 20 + j]; } std::cout << std::endl; } std::cout << "Nonce: " << *nonce << std::endl;
// Free memory delete[] out; delete nonce; cudaFree(dev_pattern); cudaFree(dev_out); cudaFree(dev_nonce);
return 0; }
blockIdx.x * blockDim.x + threadIdx.x undefinited why  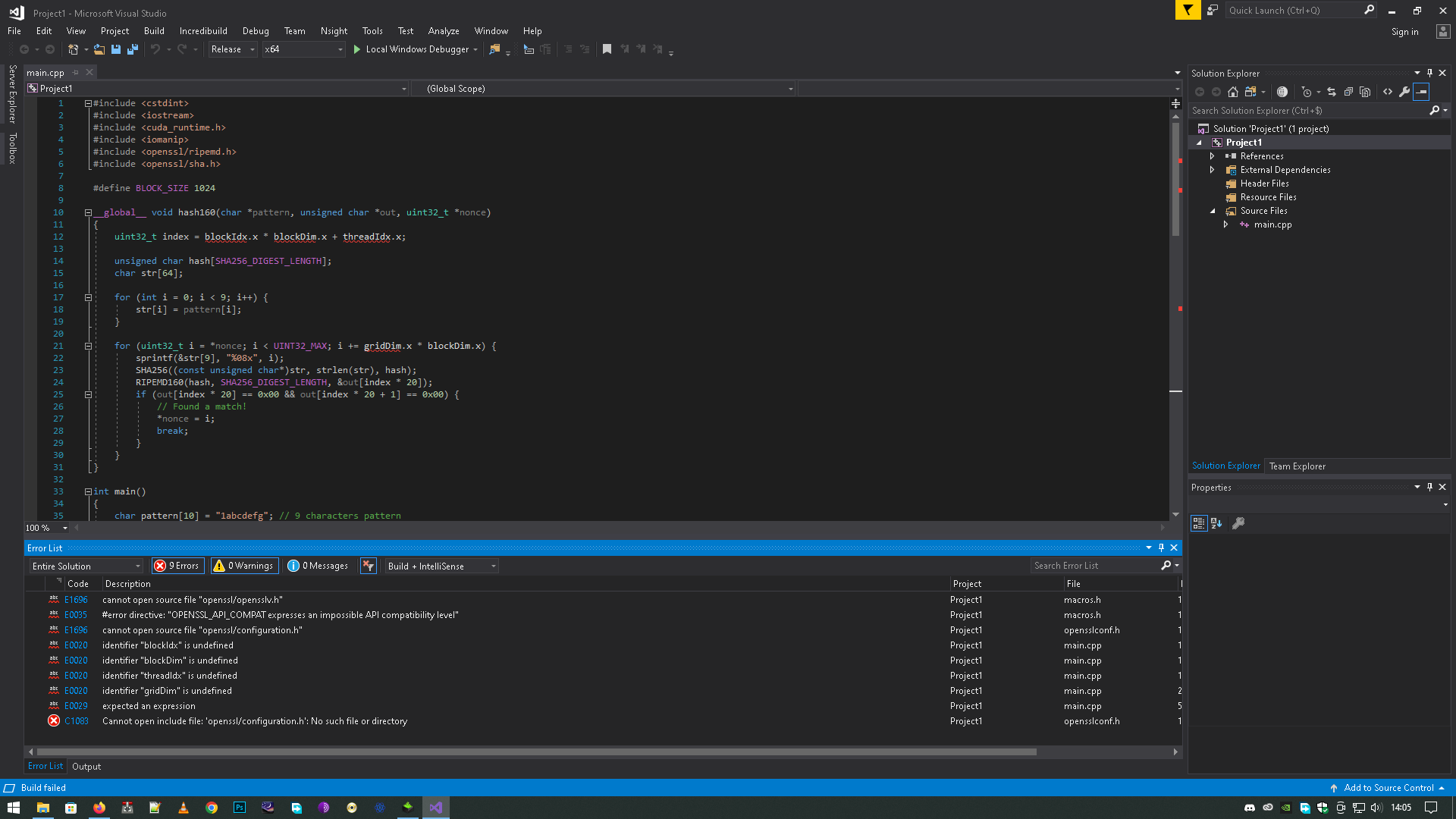
|
|
|
|
|
|
|
"If you don't want people to know you're a scumbag then don't be a scumbag." -- margaritahuyan
|
|
|
Advertised sites are not endorsed by the Bitcoin Forum. They may be unsafe, untrustworthy, or illegal in your jurisdiction.
|
|
|
|
ymgve2
|
 |
February 27, 2023, 03:00:22 PM Merited by vapourminer (1) |
|
Those are probably just warnings because Visual Studio doesn't always know about internal CUDA variables. The main issue is that it can't find your OpenSSL header file, that's what's blocking the compile.
|
|
|
|
Kostelooscoin (OP)
Member

Offline
Activity: 202
Merit: 16
|
 |
February 27, 2023, 03:04:06 PM |
|
I installed openssl but nothing works
|
|
|
|
albert0bsd
|
Where that code come from, seems that you only copy and paste part of some code. The variables that you are refering don't exist: uint32_t index = blockIdx.x * blockDim.x + threadIdx.x;
blockIdx, blockDim and threadIdx aren't declared anywhere. Regards!
|
|
|
|
Kostelooscoin (OP)
Member

Offline
Activity: 202
Merit: 16
|
 |
February 27, 2023, 04:21:28 PM |
|
I solved everything but there is still one small problem hash160 << < 3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce); in red probleme 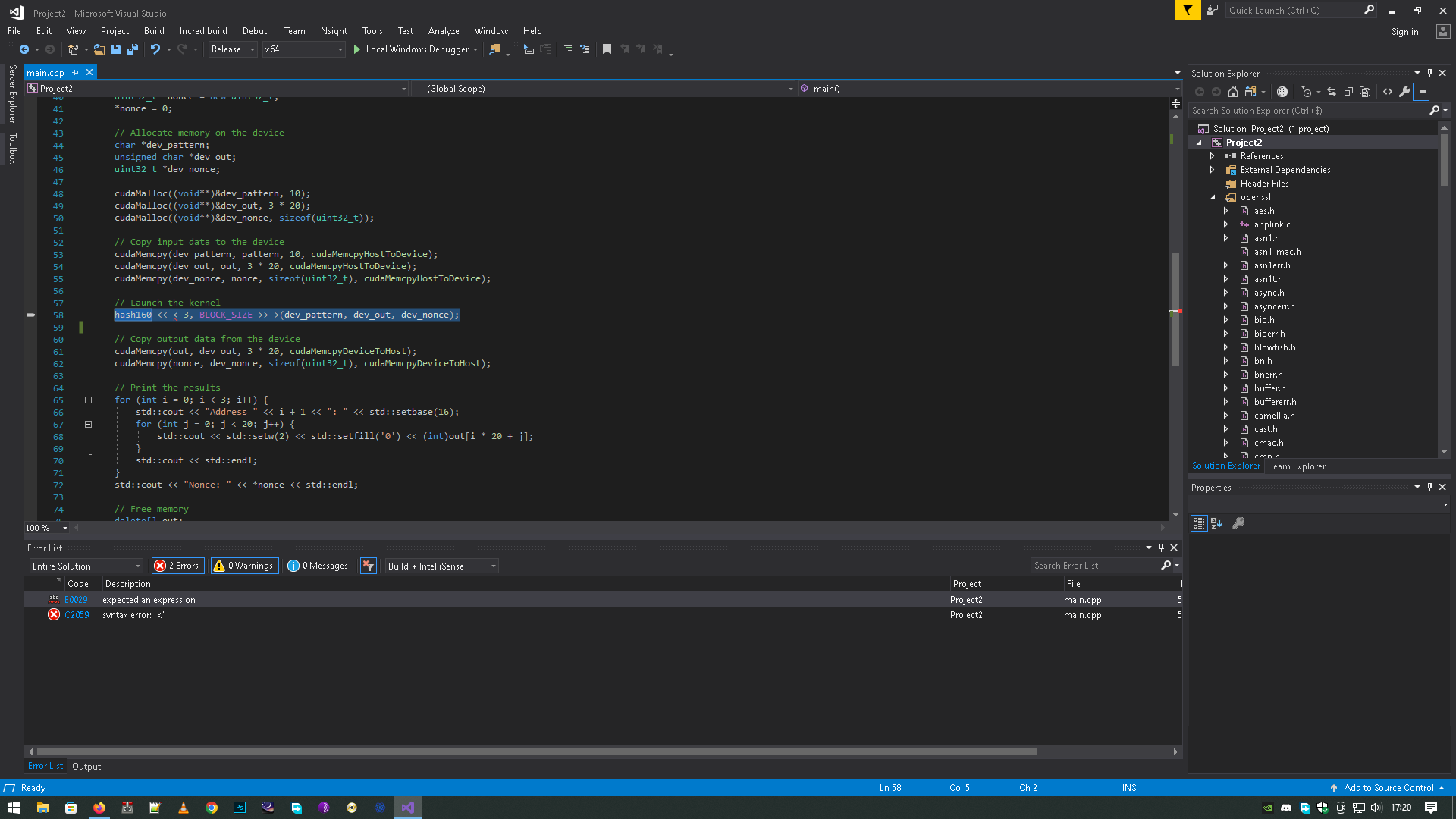
|
|
|
|
ymgve2
|
 |
February 27, 2023, 06:01:50 PM |
|
When you created the project, did you create it as a CUDA project so it uses the CUDA compiler?
|
|
|
|
Kostelooscoin (OP)
Member

Offline
Activity: 202
Merit: 16
|
 |
February 27, 2023, 06:17:02 PM |
|
yes but it doesn't change anything
|
|
|
|
NeuroticFish
Legendary
Offline
Activity: 3654
Merit: 6371
Looking for campaign manager? Contact icopress!
|
 |
February 27, 2023, 09:03:50 PM Last edit: February 27, 2023, 10:02:01 PM by NeuroticFish |
|
I solved everything but there is still one small problem
hash160 << < 3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce);
<<< works in java, not in C++ << < needs something in between (a bitwise shift maybe and then a comparison?)
But, since in the first post we have
__global__ void hash160(char *pattern, unsigned char *out, uint32_t *nonce)
you seem to be calling that void function, hence I would comment that erroneous line (at least for now) and put instead:
hash160(dev_pattern, dev_out, dev_nonce);
It seems that I was wrong, sorry, see below.
|
. .HUGE. | | | | | | █▀▀▀▀ █ █ █ █ █ █ █ █ █ █ █ █▄▄▄▄ | ▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀ . CASINO & SPORTSBOOK ▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄ | ▀▀▀▀█ █ █ █ █ █ █ █ █ █ █ █ ▄▄▄▄█ | | |
|
|
|
ymgve2
|
 |
February 27, 2023, 09:42:03 PM |
|
I solved everything but there is still one small problem
hash160 << < 3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce);
<<< works in java, not in C++ << < needs something in between (a bitwise shift maybe and then a comparison?) But, since in the first post we have __global__ void hash160(char *pattern, unsigned char *out, uint32_t *nonce) you seem to be calling that void function, hence I would comment that erroneous line (at least for now) and put instead: hash160(dev_pattern, dev_out, dev_nonce); I have difficulties to understand what you aim for with a code you cannot even read. <<<a,b>>> is an extension to C++ to launch CUDA kernels, it is perfectly valid in CUDA code https://medium.com/analytics-vidhya/cuda-compute-unified-device-architecture-part-2-f3841c25375e
|
|
|
|
NeuroticFish
Legendary
Offline
Activity: 3654
Merit: 6371
Looking for campaign manager? Contact icopress!
|
 |
February 27, 2023, 09:59:43 PM |
|
CUDA I don't know  So the only error is that extra space and should have been hash160 <<< 3, BLOCK_SIZE >>>(dev_pattern, dev_out, dev_nonce); ?? Or also Visual Studio doesn't handle this well or is missing something? That Medium page also tells (yeah, that guy was installing on Linux) about CUDA Toolkit and so on.
|
. .HUGE. | | | | | | █▀▀▀▀ █ █ █ █ █ █ █ █ █ █ █ █▄▄▄▄ | ▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀ . CASINO & SPORTSBOOK ▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄ | ▀▀▀▀█ █ █ █ █ █ █ █ █ █ █ █ ▄▄▄▄█ | | |
|
|
|
ymgve2
|
CUDA I don't know  So the only error is that extra space and should have been hash160 <<< 3, BLOCK_SIZE >>>(dev_pattern, dev_out, dev_nonce); ?? Or also Visual Studio doesn't handle this well or is missing something? That Medium page also tells (yeah, that guy was installing on Linux) about CUDA Toolkit and so on. The space doesn't matter, Visual Studio autocorrects it to << <a, b >> > and it will still compile fine if everything's set up correctly.
|
|
|
|
Kostelooscoin (OP)
Member

Offline
Activity: 202
Merit: 16
|
 |
February 28, 2023, 09:22:41 AM |
|
hash160 << <3, BLOCK_SIZE >> >(dev_pattern, dev_out, dev_nonce);
same error
|
|
|
|
|