dextronomous
|
 |
March 28, 2021, 12:51:05 AM |
|
# Blind Birthday Attack. Proof of concept. # https://defuse.ca/blind-birthday-attack.htm
require 'securerandom' require 'openssl'
# Use 32-bit HMAC so that it's actually possible to perform the attack in # a reasonable amount of time. This value must be a multiple of 8. HMAC_BITS = 32
# Compute HMAC-SHA256 def hmac(key, message) return OpenSSL::HMAC::digest('SHA256', key, message)[0, HMAC_BITS/8] end
# Here's the oracle, which we are attackking. t has a 256-bit random secret # key. When we give it two inputs a and b, it HMACs them both, and tells us how # much of the HMACs match. KEY = SecureRandom.random_bytes(32) def oracle(a, b) h1 = hmac(KEY, a) h2 = hmac(KEY, b) h1_binary = h1.bytes.map { |c| c.to_s(2).rjust(8,'0') }.join('').split('') h2_binary = h2.bytes.map { |c| c.to_s(2).rjust(8,'0') }.join('').split('') 0.upto(HMAC_BITS - 1) do |i| if h1_binary[i] != h2_binary[i] return i end end return HMAC_BITS end
# Here's an implementation of the attack.
# We organize messages into a tree to find collisions. class TreeNode attr_accessor :message, :left, :right end
# We need a good supply of unique messages. def random return SecureRandom.random_bytes(32) end
def attack # Start the tree with one random message. root = TreeNode.new root.message = random()
# Keep track of how much resources we use for the attack. queries = 0 tree_size = 0 closest = 0
# Each time this outer loop iterates, a new message is added to the tree. loop do
# Generate a new random message to add to the tree. newnode = TreeNode.new newnode.message = random()
# Start inserting at the root of the tree. current = root
# Keep track of how deep we are into the tree. # This is the number of HMAC bits that are known to match between the # 'current' node's message and the newnode's message. matching = 0
# Each time this inner loop iterates, we go one level deeper into the tree. loop do
# Ask the oracle how many bits the two HMACs match. thismatch = oracle(current.message, newnode.message) queries += 1
# If we have a better match than ever before, tell the user, so that they # don't get bored and quit the attack before it finishes. if thismatch > closest closest = thismatch puts "Closest collision so far: #{thismatch}" puts "Tree size: #{tree_size}" end
# If we found a collision, tell the user and stop the attack. if thismatch == HMAC_BITS && current.message != newnode.message puts "Found a collision amongst #{tree_size} in #{queries} queries!" puts "Message 1: #{current.message.unpack("H*")[0]}" puts "Message 2: #{newnode.message.unpack("H*")[0]}" return end
# If the (matching+1)st HMAC bit matches, we go right. Else, left. if thismatch > matching # If the right node is nil, this is where we add the new node. if current.right.nil? current.right = newnode tree_size += 1 break end # Otherwise, just move on to the next level. current = current.right else # If the left node is nil, this is where we add the new node. if current.left.nil? current.left = newnode tree_size += 1 break end # Otherwise, just move on to the next level. current = current.left end
# We've moved down to the next level. matching += 1 end
end end
attack() i found this blindbirthday thingie is this a bit what you meant andzhig
|
|
|
|
|
|
|
|
Be very wary of relying on JavaScript for security on crypto sites. The site can change the JavaScript at any time unless you take unusual precautions, and browsers are not generally known for their airtight security.
|
|
|
Advertised sites are not endorsed by the Bitcoin Forum. They may be unsafe, untrustworthy, or illegal in your jurisdiction.
|
|
|
CanalGanheAgora
Newbie
Offline
Activity: 24
Merit: 0
|
 |
March 28, 2021, 07:40:06 PM |
|
Hello guys! is there a program that saves all found addresses in a txt file? for example from one point to another: 8147ae147ae147ae:828f5c28f5c28f5c
|
|
|
|
WanderingPhilospher
Full Member
 
Offline
Activity: 1050
Merit: 219
Shooters Shoot...
|
 |
March 28, 2021, 08:37:52 PM |
|
Hello guys! is there a program that saves all found addresses in a txt file? for example from one point to another: 8147ae147ae147ae:828f5c28f5c28f5c
Just create a sequential order script to start at priv key a and end at priv key z.
|
|
|
|
CanalGanheAgora
Newbie
Offline
Activity: 24
Merit: 0
|
 |
March 29, 2021, 01:55:45 AM |
|
Hello guys! is there a program that saves all found addresses in a txt file? for example from one point to another: 8147ae147ae147ae:828f5c28f5c28f5c
Just create a sequential order script to start at priv key a and end at priv key z. where do we find a free script that does it all and still saves it in a txt file?
|
|
|
|
as9ardia
|
 |
March 30, 2021, 10:12:04 AM |
|
where do we find a free script that does it all and still saves it in a txt file?
There are several commands from various programming languages that you can use. For example, if you are using python, you can use the open() function + parameter ( w)rite open ('youfile.txt', ' w') whether your file exists or not (if the file does not exist it will create automatically) or you can use ( a)ppend parameter open ('youfile.txt', ' a') your result will be added to the existing "text" on your file
|
///Back from hiatus//// Say F to Virus!!!
|
|
|
Homeless_PhD
Newbie
Offline
Activity: 9
Merit: 1
|
 |
March 30, 2021, 10:08:19 PM |
|
where do we find a free script that does it all and still saves it in a txt file?
Look at my scripts (on github) - you may find there a way to generate 0x16 priv keys saving them into files. (i've used MATLAB/Octave)
|
|
|
|
Andzhig
Jr. Member
Offline
Activity: 183
Merit: 3
|
 |
March 31, 2021, 08:43:15 AM Last edit: March 31, 2021, 03:58:25 PM by Andzhig |
|
# Blind Birthday Attack. Proof of concept. # https://defuse.ca/blind-birthday-attack.htm
require 'securerandom' require 'openssl'
# Use 32-bit HMAC so that it's actually possible to perform the attack in # a reasonable amount of time. This value must be a multiple of 8. HMAC_BITS = 32
# Compute HMAC-SHA256 def hmac(key, message) return OpenSSL::HMAC::digest('SHA256', key, message)[0, HMAC_BITS/8] end
# Here's the oracle, which we are attackking. t has a 256-bit random secret # key. When we give it two inputs a and b, it HMACs them both, and tells us how # much of the HMACs match. KEY = SecureRandom.random_bytes(32) def oracle(a, b) h1 = hmac(KEY, a) h2 = hmac(KEY, b) h1_binary = h1.bytes.map { |c| c.to_s(2).rjust(8,'0') }.join('').split('') h2_binary = h2.bytes.map { |c| c.to_s(2).rjust(8,'0') }.join('').split('') 0.upto(HMAC_BITS - 1) do |i| if h1_binary[i] != h2_binary[i] return i end end return HMAC_BITS end
# Here's an implementation of the attack.
# We organize messages into a tree to find collisions. class TreeNode attr_accessor :message, :left, :right end
# We need a good supply of unique messages. def random return SecureRandom.random_bytes(32) end
def attack # Start the tree with one random message. root = TreeNode.new root.message = random()
# Keep track of how much resources we use for the attack. queries = 0 tree_size = 0 closest = 0
# Each time this outer loop iterates, a new message is added to the tree. loop do
# Generate a new random message to add to the tree. newnode = TreeNode.new newnode.message = random()
# Start inserting at the root of the tree. current = root
# Keep track of how deep we are into the tree. # This is the number of HMAC bits that are known to match between the # 'current' node's message and the newnode's message. matching = 0
# Each time this inner loop iterates, we go one level deeper into the tree. loop do
# Ask the oracle how many bits the two HMACs match. thismatch = oracle(current.message, newnode.message) queries += 1
# If we have a better match than ever before, tell the user, so that they # don't get bored and quit the attack before it finishes. if thismatch > closest closest = thismatch puts "Closest collision so far: #{thismatch}" puts "Tree size: #{tree_size}" end
# If we found a collision, tell the user and stop the attack. if thismatch == HMAC_BITS && current.message != newnode.message puts "Found a collision amongst #{tree_size} in #{queries} queries!" puts "Message 1: #{current.message.unpack("H*")[0]}" puts "Message 2: #{newnode.message.unpack("H*")[0]}" return end
# If the (matching+1)st HMAC bit matches, we go right. Else, left. if thismatch > matching # If the right node is nil, this is where we add the new node. if current.right.nil? current.right = newnode tree_size += 1 break end # Otherwise, just move on to the next level. current = current.right else # If the left node is nil, this is where we add the new node. if current.left.nil? current.left = newnode tree_size += 1 break end # Otherwise, just move on to the next level. current = current.left end
# We've moved down to the next level. matching += 1 end
end end
attack() i found this blindbirthday thingie is this a bit what you meant andzhig At least now it is clear how it may be called, blindbirthday https://static.wikia.nocookie.net/vikingstv/images/2/21/Seerseason3.jpg/revision/latest?cb=20150307192155 *** What if... Python and other languages use some numbers for generating random and they can be defined fixed seed() > seed(1) > seed(2) etc. and only 54 bits space (in python, "Python uses the Mersenne Twister to generate the floats. It produces 53-bit precision floats with a period of 2**19937-1.") And what if, just by passing these 54 bit can find all the puzzles (with already originated in the right order parts that need to make everything to the str and translate into dec. What is the difference that the creation of a puzzle use some kind of random with a trimming of 256 bits, from this does not change... This is the same brutfors... This seed affects the sample from the set and the mixing itself already selected. For example, when seed 0.3698697851973588, he finds a set (disordered) for 7257509 steps 0.3698697851973588 steps 7257509 ['00', '01', '02', '03', '04', '05', '06', '07', '08', '09', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24', '25', '26', '27', '28', '29', '30', '31', '32', '33', '34', '35', '36', '37', '38', '39', '40', '41', '42', '43', '44', '45', '46', '47', '48', '49', '50', '51', '52', '53', '54', '55', '56', '57', '58', '59', '60', '61', '62', '63', '64', '65', '66', '67', '68', '69', '70', '71', '72', '73', '74', '75', '76', '77', '78', '79', '80', '81', '82', '83', '84', '85', '86', '87', '88', '89', '90', '91', '92', '93', '94', '95', '96', '97', '98', '99'] 100 > ['77', '56', '31', '72', '20', '83', '03', '28', '62', '11', '88', '89', '64', '33', '30', '55', '79'] 17 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] With others can one hundred million steps run... how it is back to an integer? 0.3698697851973588 Some kind of hashing is used there https://docs.python.org/3/using/cmdline.html#envvar-PYTHONHASHSEED It needs to somehow disable to see that he ships there. upd: clear, random.random() nothing to do with it ,there is some random.getstate() who squeezes a bunch of numbers of which we have no acceptance of information not get some number... https://stackoverflow.com/questions/32172054/how-can-i-retrieve-the-current-seed-of-numpys-random-number-generatorseed(126865379) steb by step 0.5471335028603342 126865379 ['00', '01', '02', '03', '04', '05', '06', '07', '08', '09', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24', '25', '26', '27', '28', '29', '30', '31', '32', '33', '34', '35', '36', '37', '38', '39', '40', '41', '42', '43', '44', '45', '46', '47', '48', '49', '50', '51', '52', '53', '54', '55', '56', '57', '58', '59', '60', '61', '62', '63', '64', '65', '66', '67', '68', '69', '70', '71', '72', '73', '74', '75', '76', '77', '78', '79', '80', '81', '82', '83', '84', '85', '86', '87', '88', '89', '90', '91', '92', '93', '94', '95', '96', '97', '98', '99'] 100 ['23', '43', '02', '83', '30', '56', '55', '43', '77', '50', '28', '98', '31', '28', '64', '58', '20'] 17 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] Or look for parts, but when step by step substitution (from seed(1) to seed(1000000000)) seed steps 145448653 ['31', '56', '77', '83', '30'] 5 ['30'] ['56'] ['83'] ['77'] ['31'] In any case, this can be controlled by the set. random.seed(12) loop 1 loop 2 0 loop 3 0 1 loop 1 loop 2 0 loop 3 0 2 loop 1 loop 2 0 loop 3 0 3 loop 1 loop 2 0 loop 3 0 4 loop 1 loop 2 0 loop 3 0 5 loop 1 6 huuurrraaa... ['18', '40', '07', '08', '57', '81', '20', '54', '55', '03', '58', '82', '40', '28', '09', '47', '65', '92', '60', '76', '18', '05', '65', '06', '27', '77', '62', '83', '01', '58', '38', '78', '78', '64', '32', '22', '09', '93', '50', '26', '05', '56', '26', '58', '21', '81', '75', '75', '01', '66', '30', '57', '08', '30', '85', '78', '00', '21', '12', '31'] 60 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] loop 2 1 loop 3 0 6 loop 1 100 huuurrraaa... ['30', '18', '31', '00', '12', '60', '10', '33', '17', '84', '13', '55', '52', '13', '65', '98', '70', '19', '47', '46', '52', '55', '52', '36', '91', '48', '76', '56', '04', '11', '64', '54', '48', '27', '53', '81', '56', '49', '34', '21', '28', '36', '46', '33', '72', '58', '59', '83', '53', '39', '77', '77', '20', '26', '35', '42', '23', '76', '44', '36'] 60 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] loop 2 1 12 huuurrraaa... ['19', '47', '64', '55', '84', '77', '56', '36', '84', '31', '56', '30', '52', '48', '28', '55', '76', '19', '47', '76', '20', '11', '49', '70', '83', '26', '35', '56', '47', '83'] 30 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] loop 3 1 [100] [12] 1 88 huuurrraaa... ['36', '77', '36', '47', '55', '30', '47', '47', '20', '77', '28', '83', '56', '31', '20', '83', '64'] 17 ['30'] ['56'] ['83'] ['77'] ['31'] ['20'] ['64'] ['20'] ['28'] ['55'] 100 100 500 on 7 loop (100x100x6=60000 from 100 to 12 to 88)
|
|
|
|
matan
Member

Offline
Activity: 99
Merit: 10
|
 |
March 31, 2021, 02:30:27 PM |
|
The puzzle was solved, wasn't it? Did someone get a prize?
|
|
|
|
|
CanalGanheAgora
Newbie
Offline
Activity: 24
Merit: 0
|
 |
April 01, 2021, 11:29:57 PM |
|
This puzzle is very strange. If it's for measuring the world's brute forcing capacity, 161-256 are just a waste (RIPEMD160 entropy is filled by 160, and by all of P2PKH Bitcoin). The puzzle creator could improve the puzzle's utility without bringing in any extra funds from outside - just spend 161-256 across to the unsolved portion 51-160, and roughly treble the puzzle's content density. If on the other hand there's a pattern to find... well... that's awfully open-ended... can we have a hint or two?  I am the creator. You are quite right, 161-256 are silly. I honestly just did not think of this. What is especially embarrassing, is this did not occur to me once, in two years. By way of excuse, I was not really thinking much about the puzzle at all. I will make up for two years of stupidity. I will spend from 161-256 to the unsolved parts, as you suggest. In addition, I intend to add further funds. My aim is to boost the density by a factor of 10, from 0.001*length(key) to 0.01*length(key). Probably in the next few weeks. At any rate, when I next have an extended period of quiet and calm, to construct the new transaction carefully. A few words about the puzzle. There is no pattern. It is just consecutive keys from a deterministic wallet (masked with leading 000...0001 to set difficulty). It is simply a crude measuring instrument, of the cracking strength of the community. Finally, I wish to express appreciation of the efforts of all developers of new cracking tools and technology. The "large bitcoin collider" is especially innovative and interesting! Please if it is possible for us to release the pubkeys from the other addresses that are missing. We are grateful! United We Are One! Donate: 1C6wmXbktMA1peHMDbDRShAhJ6Wd8Mypw8
|
|
|
|
Andzhig
Jr. Member
Offline
Activity: 183
Merit: 3
|
 |
April 02, 2021, 07:36:28 PM |
|
This puzzle is very strange. If it's for measuring the world's brute forcing capacity, 161-256 are just a waste (RIPEMD160 entropy is filled by 160, and by all of P2PKH Bitcoin). The puzzle creator could improve the puzzle's utility without bringing in any extra funds from outside - just spend 161-256 across to the unsolved portion 51-160, and roughly treble the puzzle's content density. If on the other hand there's a pattern to find... well... that's awfully open-ended... can we have a hint or two?  I am the creator. You are quite right, 161-256 are silly. I honestly just did not think of this. What is especially embarrassing, is this did not occur to me once, in two years. By way of excuse, I was not really thinking much about the puzzle at all. I will make up for two years of stupidity. I will spend from 161-256 to the unsolved parts, as you suggest. In addition, I intend to add further funds. My aim is to boost the density by a factor of 10, from 0.001*length(key) to 0.01*length(key). Probably in the next few weeks. At any rate, when I next have an extended period of quiet and calm, to construct the new transaction carefully. A few words about the puzzle. There is no pattern. It is just consecutive keys from a deterministic wallet (masked with leading 000...0001 to set difficulty). It is simply a crude measuring instrument, of the cracking strength of the community. Finally, I wish to express appreciation of the efforts of all developers of new cracking tools and technology. The "large bitcoin collider" is especially innovative and interesting! Please if it is possible for us to release the pubkeys from the other addresses that are missing. We are grateful! United We Are One! Donate: 1C6wmXbktMA1peHMDbDRShAhJ6Wd8Mypw8 What for. You still do not have time to catch anything faster others with rigs. All stay at 120 bits. *** Continuation of the blind blindbirthday... Choose (random.choice 2x) from a set (00-99) with a fixed seed() Find seed(3435) 0000 seed(108918) 0001 seed(4327) 0002 etc.. from 0000 to 9999 10000 similarly 10000 different seed 3435, 108918, 4327, 20723, 9991, 10068, 8175, 10495, 23048, 5511, 3085 etc... seed [ 2532, 679, 4652, 2530, 620] pz 3056 8377 3120 6420 2855 But we have an unlimited number of numbers for fixed results seed 2532 pz 3056 seed 3801 pz 3056 seed 4476 pz 3056 seed 6363 pz 3056 etc... seed 1000000000974 pz 3056 seed 1000000006200 pz 3056 seed 1000000072946 pz 3056 seed 1000000074588 pz 3056 etc... Are there patterns in the units of steps seed or rand?... But as in the case of a black hole (2^...) Here is a wormhole When increasing the number pz will grow and seed seed 3056 seeed 30568 seeeeed 305683 seeeeeeed 3056837 seeeeeeeed 30568377 etc and these seed for numbers 10 or 20 digits long too repeated. If we choose 2 samples for 4 len num by 1 seed, we can choose by 1 seed from 10 num len set 0000000000-9999999999 for pz 20 len (the question is how much it will occupy the ram of the system resources)
|
|
|
|
CanalGanheAgora
Newbie
Offline
Activity: 24
Merit: 0
|
 |
April 03, 2021, 05:05:30 AM |
|
This puzzle is very strange. If it's for measuring the world's brute forcing capacity, 161-256 are just a waste (RIPEMD160 entropy is filled by 160, and by all of P2PKH Bitcoin). The puzzle creator could improve the puzzle's utility without bringing in any extra funds from outside - just spend 161-256 across to the unsolved portion 51-160, and roughly treble the puzzle's content density. If on the other hand there's a pattern to find... well... that's awfully open-ended... can we have a hint or two?  I am the creator. You are quite right, 161-256 are silly. I honestly just did not think of this. What is especially embarrassing, is this did not occur to me once, in two years. By way of excuse, I was not really thinking much about the puzzle at all. I will make up for two years of stupidity. I will spend from 161-256 to the unsolved parts, as you suggest. In addition, I intend to add further funds. My aim is to boost the density by a factor of 10, from 0.001*length(key) to 0.01*length(key). Probably in the next few weeks. At any rate, when I next have an extended period of quiet and calm, to construct the new transaction carefully. A few words about the puzzle. There is no pattern. It is just consecutive keys from a deterministic wallet (masked with leading 000...0001 to set difficulty). It is simply a crude measuring instrument, of the cracking strength of the community. Finally, I wish to express appreciation of the efforts of all developers of new cracking tools and technology. The "large bitcoin collider" is especially innovative and interesting! Please if it is possible for us to release the pubkeys from the other addresses that are missing. We are grateful! United We Are One! Donate: 1C6wmXbktMA1peHMDbDRShAhJ6Wd8Mypw8 What for. You still do not have time to catch anything faster others with rigs. All stay at 120 bits. *** Continuation of the blind blindbirthday... Choose (random.choice 2x) from a set (00-99) with a fixed seed() Find seed(3435) 0000 seed(108918) 0001 seed(4327) 0002 etc.. from 0000 to 9999 10000 similarly 10000 different seed 3435, 108918, 4327, 20723, 9991, 10068, 8175, 10495, 23048, 5511, 3085 etc... seed [ 2532, 679, 4652, 2530, 620] pz 3056 8377 3120 6420 2855 But we have an unlimited number of numbers for fixed results seed 2532 pz 3056 seed 3801 pz 3056 seed 4476 pz 3056 seed 6363 pz 3056 etc... seed 1000000000974 pz 3056 seed 1000000006200 pz 3056 seed 1000000072946 pz 3056 seed 1000000074588 pz 3056 etc... Are there patterns in the units of steps seed or rand?... But as in the case of a black hole (2^...) Here is a wormhole When increasing the number pz will grow and seed seed 3056 seeed 30568 seeeeed 305683 seeeeeeed 3056837 seeeeeeeed 30568377 etc and these seed for numbers 10 or 20 digits long too repeated. If we choose 2 samples for 4 len num by 1 seed, we can choose by 1 seed from 10 num len set 0000000000-9999999999 for pz 20 len (the question is how much it will occupy the ram of the system resources) How to save in a text file all the pubkey passed until reaching the pubkey of origin? Start: 8000000000000000 End: ffffffffffffffff Pubkey: 0330de2c8bc2010aaebbb647c5bac00eb8028f78d795f2cd4532bc6c504c0e01e7 how to save all MK / s FOUND to a txt file? check out the image: https://i.ibb.co/hsZ04dv/Screenshot-3.png
|
|
|
|
WanderingPhilospher
Full Member
 
Offline
Activity: 1050
Merit: 219
Shooters Shoot...
|
 |
April 03, 2021, 05:19:55 AM |
|
How to save in a text file all the pubkey passed until reaching the pubkey of origin?
Start: 8000000000000000 End: ffffffffffffffff
Pubkey: 0330de2c8bc2010aaebbb647c5bac00eb8028f78d795f2cd4532bc6c504c0e01e7
how to save all MK / s FOUND to a txt file? Use the -wi and -w option to save your work and then export your saved file and it will be in .txt format
|
|
|
|
fxsniper
Member

Offline
Activity: 406
Merit: 45
|
 |
April 03, 2021, 08:44:11 AM |
|
How to save in a text file all the pubkey passed until reaching the pubkey of origin? Start: 8000000000000000 End: ffffffffffffffff Pubkey: 0330de2c8bc2010aaebbb647c5bac00eb8028f78d795f2cd4532bc6c504c0e01e7 how to save all MK / s FOUND to a txt file? check out the image: 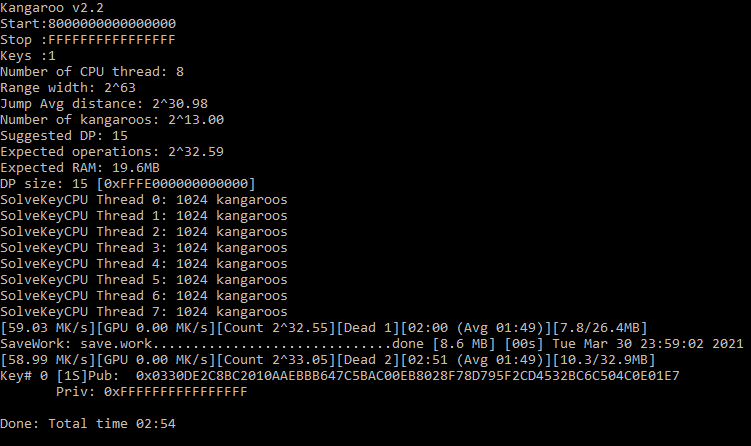 Pubkey: 0330de2c8bc2010aaebbb647c5bac00eb8028f78d795f2cd4532bc6c504c0e01e7 This is testing right -o fileName: output result to fileName use option -o output.txt or -o results.txt (any name) to save key when found the key
|
|
|
|
Andzhig
Jr. Member
Offline
Activity: 183
Merit: 3
|
 |
April 05, 2021, 07:27:20 AM |
|
Well, they can even end in small diquses seed (1, 10**5000) 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 000000000000000000000000000000000000000000000000000000000000000000000009 3056 ['30', '56'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 007 3056 ['30', '56'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 000000002 3056 ['30', '56'] 2
***
1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000000000000000000000000007 8377 ['83', '77'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000000000000 8377 ['83', '77'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000000000000000000000000000000000000000000000000000000000000000003 8377 ['83', '77'] 2
***
1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000008 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000004 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000004 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000006 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000000000000000000000000000000000000000000006 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 000000000000000000000000000000000000000000000000000008 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000000000001 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 00000000001 3120 ['31', '20'] 2 1000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000005 3120 ['31', '20'] 2etc... End of 0 to 10 for example But grows threshold 10^... wonder if there could be such 10^... Ending from 0 to 10 for 20 digit numbers... For 8 digits 30568377 seed() 1000000000000344911724 1000000000000365965249 1000000000000446475347 1000000000000586845372 1000000000000601318172 1000000000000736794481 1000000000000785724585 1000000000000942254030 1000000000000999458354 for 10 digit fishing 3056837731 seed (0, 3107724435) 2064202855 seed (0, 1487544924) for 20 digits haven't caught yet , probably, they are also long 20 or 21 22 characters or see the beginning of the message... Also possible search option (anatted for GPU) Initiate through seed() sampling of sapper shovel for example for 5,6,7 sets seed(10561492) seed(30206027) seed(2212748134) for 10, I have not found yet... import random from bit import Key import time import itertools
for X in range(1,10000000000000): R=X #print(R) random.seed(R)
list = ["16jY7qLJnxb7CHZyqBP8qca9d51gAjyXQN","13zb1hQbWVsc2S7ZTZnP2G4undNNpdh5so","1BY8GQbnueYofwSuFAT3USAhGjPrkxDdW9","18ZMbwUFLMHoZBbfpCjUJQTCMCbktshgpe"]
#1 2 3 4 5 6 7 8 9 10
a0= [(0, 0)] # 1 a1= [(1, 0), (0, 1)] # 2 a2= [(2, 0), (1, 1), (0, 2)] # 3 a3= [(3, 0), (2, 1), (1, 2), (0, 3)] # 4 a4= [(4, 0), (3, 1), (2, 2), (1, 3), (0, 4)] # 5 a5= [(5, 0), (4, 1), (3, 2), (2, 3), (1, 4), (0, 5)] # 6 a6= [(6, 0), (5, 1), (4, 2), (3, 3), (2, 4), (1, 5), (0, 6)] # 7 a7= [(7, 0), (6, 1), (5, 2), (4, 3), (3, 4), (2, 5), (1, 6), (0, 7)] # 8 a8= [(8, 0), (7, 1), (6, 2), (5, 3), (4, 4), (3, 5), (2, 6), (1, 7), (0, 8 )] # 9 a9= [(9, 0), (8, 1), (7, 2), (6, 3), (5, 4), (4, 5), (3, 6), (2, 7), (1, 8 ), (0, 9)] # 10 a10= [(9, 1), (8, 2), (7, 3), (6, 4), (5, 5), (4, 6), (3, 7), (2, 8 ), (1, 9)] # 9 a11= [(9, 2), (8, 3), (7, 4), (6, 5), (5, 6), (4, 7), (3, 8 ), (2, 9)] # 8 a12= [(9, 3), (8, 4), (7, 5), (6, 6), (5, 7), (4, 8 ), (3, 9)] # 7 a13= [(9, 4), (8, 5), (7, 6), (6, 7), (5, 8 ), (4, 9)] # 6 a14= [(9, 5), (8, 6), (7, 7), (6, 8 ), (5, 9)] # 5 a15= [(9, 6), (8, 7), (7, 8 ), (6, 9)] # 4 a16= [(9, 7), (8, 8 ), (7, 9)] # 3 a17= [(9, 8 ), (8, 9)] # 2 a18= [(9, 9)] # 1
while True:
v1 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) #[a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18] v2 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v3 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v4 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v5 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v6 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v7 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v8 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v9 = random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18]) v10= random.choice([a0,a1,a2,a3,a4,a5,a6,a7,a8,a9,a10,a11,a12,a13,a14,a15,a16,a17,a18])
if v1 == a3: if v2 == a11: if v3 == a11: if v4 == a14: if v5 == a4: if v6 == a2: if v7 == a10: if v8 == a2: if v9 == a10: if v10 == a10:
print(len(v1),v1) print(len(v2),v2) print(len(v3),v3) print(len(v4),v4) print(len(v5),v5) print(len(v6),v6) print(len(v7),v7) print(len(v8),v8) print(len(v9),v9) print(len(v10),v10)
ur = len(v1)*len(v2)*len(v3)*len(v4)*len(v5)*len(v6)*len(v7)*len(v8)*len(v9)*len(v10) print(len(v1),len(v2),len(v3),len(v4),len(v5),len(v6),len(v7),len(v8),len(v9),len(v10),"=",ur) time.sleep(3.0) count = 0 if ur >= 1000000: if ur <= 150000000: for x in itertools.product(v1, v2, v3, v4, v5, v6, v7, v8, v9, v10): count += 1 d = ("".join(map(str, (item for sublist in x for item in sublist))))
ran = int(d) key1 = Key.from_int(ran) addr1 = key1.address if addr1 in list:
print (ran,"found!!!")
s5 = str(ran) f=open(u"C:/a.txt","a") f.write(s5 + '\n') f.close()
break
else: #pass print(R,len(v1),len(v2),len(v3),len(v4),len(v5),len(v6),len(v7),len(v8),len(v9),len(v10),"=",ur,count,ran,len(d),addr1) #("all steps",lenn,count,ran,len(d),addr1)
break
|
|
|
|
fxsniper
Member

Offline
Activity: 406
Merit: 45
|
 |
April 08, 2021, 08:48:55 AM |
|
I found hack page or may be webmaster hide page (may be scam) this page random bitcoin address generator www.50pansa.info/random.htmlso, have any brute force address on java script I not sure this script is clean or not, just fine to safety file on github (however it is lowly) Can possible anybody help to modify to brute force ? it can run on mobile easy
|
|
|
|
Andzhig
Jr. Member
Offline
Activity: 183
Merit: 3
|
 |
April 08, 2021, 01:46:43 PM Last edit: April 08, 2021, 05:39:00 PM by Andzhig |
|
I found hack page or may be webmaster hide page (may be scam) this page random bitcoin address generator www.50pansa.info/random.htmlso, have any brute force address on java script I not sure this script is clean or not, just fine to safety file on github (however it is lowly) Can possible anybody help to modify to brute force ? it can run on mobile easy Recently mentioned this shit https://bitcointalk.org/index.php?topic=4768828.0 , sha256 brainwallet brut... take any information https://github.com/minimaxir/textgenrnn ,+ idioms https://7esl.com/english-idioms/#What_is_an_Idiom ,+ https://en.wikipedia.org/wiki/Chengyu convert in sha... or any other hashiers (including several times) and in search of treasures. On mobile can used "Pydroid 3 - IDE for Python 3" https://play.google.com/store/apps/details?id=ru.iiec.pydroid3&hl=en_IN but you have to look at the possibility of the device. To appeal, save it is necessary to write there "/sdcard/..." *** seed() blindbirthday search import random from bit import Key #from bit.format import bytes_to_wif #from PyRandLib import * #rand = FastRand63() #random.seed(rand()) #mp.dps = 100; mp.pretty = True import time
list = ["16jY7qLJnxb7CHZyqBP8qca9d51gAjyXQN","13zb1hQbWVsc2S7ZTZnP2G4undNNpdh5so","1BY8GQbnueYofwSuFAT3USAhGjPrkxDdW9", "1MVDYgVaSN6iKKEsbzRUAYFrYJadLYZvvZ","19vkiEajfhuZ8bs8Zu2jgmC6oqZbWqhxhG","1DJh2eHFYQfACPmrvpyWc8MSTYKh7w9eRF", "1PWo3JeB9jrGwfHDNpdGK54CRas7fsVzXU","1JTK7s9YVYywfm5XUH7RNhHJH1LshCaRFR","12VVRNPi4SJqUTsp6FmqDqY5sGosDtysn4", "1FWGcVDK3JGzCC3WtkYetULPszMaK2Jksv","1DJh2eHFYQfACPmrvpyWc8MSTYKh7w9eRF","1Bxk4CQdqL9p22JEtDfdXMsng1XacifUtE", "15qF6X51huDjqTmF9BJgxXdt1xcj46Jmhb","1ARk8HWJMn8js8tQmGUJeQHjSE7KRkn2t8","15qsCm78whspNQFydGJQk5rexzxTQopnHZ", "13zYrYhhJxp6Ui1VV7pqa5WDhNWM45ARAC","14MdEb4eFcT3MVG5sPFG4jGLuHJSnt1Dk2","1CMq3SvFcVEcpLMuuH8PUcNiqsK1oicG2D", "1K3x5L6G57Y494fDqBfrojD28UJv4s5JcK","1PxH3K1Shdjb7gSEoTX7UPDZ6SH4qGPrvq","16AbnZjZZipwHMkYKBSfswGWKDmXHjEpSf", "19QciEHbGVNY4hrhfKXmcBBCrJSBZ6TaVt","1EzVHtmbN4fs4MiNk3ppEnKKhsmXYJ4s74","1AE8NzzgKE7Yhz7BWtAcAAxiFMbPo82NB5", "17Q7tuG2JwFFU9rXVj3uZqRtioH3mx2Jad","1K6xGMUbs6ZTXBnhw1pippqwK6wjBWtNpL","15ANYzzCp5BFHcCnVFzXqyibpzgPLWaD8b", "18ywPwj39nGjqBrQJSzZVq2izR12MDpDr8","1CaBVPrwUxbQYYswu32w7Mj4HR4maNoJSX","1JWnE6p6UN7ZJBN7TtcbNDoRcjFtuDWoNL", "1CKCVdbDJasYmhswB6HKZHEAnNaDpK7W4n","1PXv28YxmYMaB8zxrKeZBW8dt2HK7RkRPX","1AcAmB6jmtU6AiEcXkmiNE9TNVPsj9DULf", "1EQJvpsmhazYCcKX5Au6AZmZKRnzarMVZu","18KsfuHuzQaBTNLASyj15hy4LuqPUo1FNB","15EJFC5ZTs9nhsdvSUeBXjLAuYq3SWaxTc", "1HB1iKUqeffnVsvQsbpC6dNi1XKbyNuqao","1GvgAXVCbA8FBjXfWiAms4ytFeJcKsoyhL","12JzYkkN76xkwvcPT6AWKZtGX6w2LAgsJg", "1824ZJQ7nKJ9QFTRBqn7z7dHV5EGpzUpH3","18A7NA9FTsnJxWgkoFfPAFbQzuQxpRtCos","1NeGn21dUDDeqFQ63xb2SpgUuXuBLA4WT4", "1NLbHuJebVwUZ1XqDjsAyfTRUPwDQbemfv","1MnJ6hdhvK37VLmqcdEwqC3iFxyWH2PHUV","1KNRfGWw7Q9Rmwsc6NT5zsdvEb9M2Wkj5Z", "1PJZPzvGX19a7twf5HyD2VvNiPdHLzm9F6","1GuBBhf61rnvRe4K8zu8vdQB3kHzwFqSy7","17s2b9ksz5y7abUm92cHwG8jEPCzK3dLnT", "1GDSuiThEV64c166LUFC9uDcVdGjqkxKyh","1Me3ASYt5JCTAK2XaC32RMeH34PdprrfDx","1CdufMQL892A69KXgv6UNBD17ywWqYpKut", "1BkkGsX9ZM6iwL3zbqs7HWBV7SvosR6m8N","1PXAyUB8ZoH3WD8n5zoAthYjN15yN5CVq5","1AWCLZAjKbV1P7AHvaPNCKiB7ZWVDMxFiz", "1G6EFyBRU86sThN3SSt3GrHu1sA7w7nzi4","1MZ2L1gFrCtkkn6DnTT2e4PFUTHw9gNwaj","1Hz3uv3nNZzBVMXLGadCucgjiCs5W9vaGz", "1Fo65aKq8s8iquMt6weF1rku1moWVEd5Ua","16zRPnT8znwq42q7XeMkZUhb1bKqgRogyy","1KrU4dHE5WrW8rhWDsTRjR21r8t3dsrS3R", "17uDfp5r4n441xkgLFmhNoSW1KWp6xVLD","13A3JrvXmvg5w9XGvyyR4JEJqiLz8ZySY3","16RGFo6hjq9ym6Pj7N5H7L1NR1rVPJyw2v", "1UDHPdovvR985NrWSkdWQDEQ1xuRiTALq","15nf31J46iLuK1ZkTnqHo7WgN5cARFK3RA","1Ab4vzG6wEQBDNQM1B2bvUz4fqXXdFk2WT", "1Fz63c775VV9fNyj25d9Xfw3YHE6sKCxbt","1QKBaU6WAeycb3DbKbLBkX7vJiaS8r42Xo","1CD91Vm97mLQvXhrnoMChhJx4TP9MaQkJo", "15MnK2jXPqTMURX4xC3h4mAZxyCcaWWEDD","13N66gCzWWHEZBxhVxG18P8wyjEWF9Yoi1","1NevxKDYuDcCh1ZMMi6ftmWwGrZKC6j7Ux", "19GpszRNUej5yYqxXoLnbZWKew3KdVLkXg","1M7ipcdYHey2Y5RZM34MBbpugghmjaV89P","18aNhurEAJsw6BAgtANpexk5ob1aGTwSeL", "1FwZXt6EpRT7Fkndzv6K4b4DFoT4trbMrV","1CXvTzR6qv8wJ7eprzUKeWxyGcHwDYP1i2","1MUJSJYtGPVGkBCTqGspnxyHahpt5Te8jy", "13Q84TNNvgcL3HJiqQPvyBb9m4hxjS3jkV","1LuUHyrQr8PKSvbcY1v1PiuGuqFjWpDumN","18192XpzzdDi2K11QVHR7td2HcPS6Qs5vg", "1NgVmsCCJaKLzGyKLFJfVequnFW9ZvnMLN","1AoeP37TmHdFh8uN72fu9AqgtLrUwcv2wJ","1FTpAbQa4h8trvhQXjXnmNhqdiGBd1oraE", "14JHoRAdmJg3XR4RjMDh6Wed6ft6hzbQe9","19z6waranEf8CcP8FqNgdwUe1QRxvUNKBG","14u4nA5sugaswb6SZgn5av2vuChdMnD9E5", "174SNxfqpdMGYy5YQcfLbSTK3MRNZEePoy", "1NBC8uXJy1GiJ6drkiZa1WuKn51ps7EPTv"] Nn = ['00', '01', '02', '03', '04', '05', '06', '07', '08', '09', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24', '25', '26', '27', '28', '29', '30', '31', '32', '33', '34', '35', '36', '37', '38', '39', '40', '41', '42', '43', '44', '45', '46', '47', '48', '49', '50', '51', '52', '53', '54', '55', '56', '57', '58', '59', '60', '61', '62', '63', '64', '65', '66', '67', '68', '69', '70', '71', '72', '73', '74', '75', '76', '77', '78', '79', '80', '81', '82', '83', '84', '85', '86', '87', '88', '89', '90', '91', '92', '93', '94', '95', '96', '97', '98', '99']
def func1(): random.seed() d = random.randint(1,100) # starting step selection ...000000001, ...000000004, ...0000000046724618237621312879 etc... return d
hhh = func1() print(hhh,"starting step selection ") print("") time.sleep(3.0)
i= 1 # starting leading zeros 10 100 1000 etc, 19 for 20 len num while i <= 10**5000: # duration of zeros ...00000000000... vvv = 10**i for X in range (0,100): # steps between adding zeros (duration of zeros)
vvv += hhh
R=vvv #print(R) random.seed(R)
RRR = [] #RRR2 = []
for RR in range(25): # set 00-99 screening out length, seed() is the same for 2 and 10, 20, 30 samples... DDD = random.choice(Nn) RRR.append(DDD)
count = 0 d = ''.join(RRR) A = 20 while A <= 50:
#time.sleep(0.02) dd = (d)[0:A] ran = int(dd) key1 = Key.from_int(ran) addr1 = key1.address if addr1 in list:
print (ran,"found!!!")
s5 = str(ran) f=open(u"C:/a.txt","a") # need to create a file in C:\a.txt ,or rewrite the code (possible problems with administrator rights) f.write(s5 + '\n') f.close() time.sleep(600.0) break
else: #pass #pass count += 1 print(vvv,count,ran,len(dd),addr1,RRR) # A=A+1
RRR=[] count = 0 #RRR2=[] #print("loop end...") #time.sleep(3.0)
i=i+1
#import time print("") print("loop end...") time.sleep(600.0)
|
|
|
|
fxsniper
Member

Offline
Activity: 406
Merit: 45
|
 |
April 08, 2021, 04:38:47 PM |
|
Thank you very much for Pydroid 3 - IDE for Python 3 very good app I know brainwallet is not safety but we can modify and change to random 256bit number right
|
|
|
|
fxsniper
Member

Offline
Activity: 406
Merit: 45
|
 |
April 21, 2021, 11:26:42 AM |
|
He almost certainly used some env with good entropy, he obviously knew what he was doing. Zero chance that these random numbers have a weakness on PRNG side.
Can entropy formula use for calculate position zone of puzzle SI unit: joules per kelvin (J⋅K−1) In SI base units: kg⋅m2⋅s−2⋅K−1 https://en.wikipedia.org/wiki/Entropymay be not find directly to key , just want to know zone or area nearly key for using bitcrack or kangaroo to fine again
|
|
|
|
takefive
Newbie
Offline
Activity: 1
Merit: 0
|
 |
April 21, 2021, 11:46:28 AM Last edit: April 24, 2021, 08:19:10 AM by takefive |
|
Whats the range for the 60th address? Need to upgrade my blind monk script bitlife pc jiofi.local.html
|
|
|
|
|