Denis_Hitov
Newbie
Offline
Activity: 49
Merit: 0
|
 |
October 12, 2023, 08:39:54 PM |
|
I don't have any error on multiple machines. Make sure that is at least python3.9 installed. I changed the order of the code a bit. If there is still an error, something is wrong with python there. import sys import os import time import random import hashlib import gmpy2 from gmpy2 import mpz from functools import lru_cache import multiprocessing from multiprocessing import Pool, cpu_count
os.system("clear")
# Constants MODULO = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEFFFFFC2F) ORDER = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEBAAEDCE6AF48A03BBFD25E8CD0364141) GX = gmpy2.mpz(0x79BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F81798) GY = gmpy2.mpz(0x483ADA7726A3C4655DA4FBFC0E1108A8FD17B448A68554199C47D08FFB10D4B8)
# Define Point class class Point: def __init__(self, x=0, y=0): self.x = x self.y = y
PG = Point(GX, GY) ZERO_POINT = Point(0, 0)
# Function to multiply a point by 2 def multiply_by_2(P, p=MODULO): c = gmpy2.f_mod(3 * P.x * P.x * gmpy2.powmod(2 * P.y, -1, p), p) R = Point() R.x = gmpy2.f_mod(c * c - 2 * P.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to add two points def add_points(P, Q, p=MODULO): dx = Q.x - P.x dy = Q.y - P.y c = gmpy2.f_mod(dy * gmpy2.invert(dx, p), p) R = Point() R.x = gmpy2.f_mod(c * c - P.x - Q.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to calculate Y-coordinate from X-coordinate @lru_cache(maxsize=None) def x_to_y(X, y_parity, p=MODULO): Y = gmpy2.mpz(3) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
X = gmpy2.f_mod(tmp + 7, p)
Y = gmpy2.f_div(gmpy2.add(p, 1), 4) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
Y = tmp
if Y % 2 != y_parity: Y = gmpy2.f_mod(-Y, p)
return Y
# Function to compute a table of points def compute_point_table(): points = [PG] for k in range(255): points.append(multiply_by_2(points[k])) return points
POINTS_TABLE = compute_point_table()
# Global event to signal all processes to stop STOP_EVENT = multiprocessing.Event()
# Function to check and compare points for potential solutions def check(P, Pindex, DP_rarity, A, Ak, B, Bk): check = gmpy2.f_mod(P.x, DP_rarity) if check == 0: message = f"\r[+] [Pindex]: {mpz(Pindex)}" messages = [] messages.append(message) output = "\033[01;33m" + ''.join(messages) + "\r" sys.stdout.write(output) sys.stdout.flush() A.append(mpz(P.x)) Ak.append(mpz(Pindex)) return comparator(A, Ak, B, Bk) else: return False
# Function to compare two sets of points and find a common point def comparator(A, Ak, B, Bk): global STOP_EVENT result = set(A).intersection(set(B))
if result: sol_kt = A.index(next(iter(result))) sol_kw = B.index(next(iter(result))) difference = Ak[sol_kt] - Bk[sol_kw] HEX = "%064x" % difference t = time.ctime() pid = os.getpid() # Get the process ID core_number = pid % cpu_count() # Calculate the CPU core number total_time = time.time() - starttime print(f"\033[32m[+] PUZZLE SOLVED: {t}, total time: {total_time:.2f} sec, Core: {core_number+1:02} \033[0m") print(f"\033[32m[+] WIF: \033[32m {HEX} \033[0m") with open("KEYFOUNDKEYFOUND.txt", "a") as file: file.write("\n\nSOLVED " + t) file.write(f"\nTotal Time: {total_time:.2f} sec") file.write("\nPrivate Key (decimal): " + str(difference)) file.write("\nPrivate Key (hex): " + HEX) file.write( "\n-------------------------------------------------------------------------------------------------------------------------------------\n" )
STOP_EVENT.set() # Set the stop event to signal all processes
# Memoization for point multiplication ECMULTIPLY_MEMO = {}
# Function to multiply a point by a scalar def ecmultiply(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: if k in ECMULTIPLY_MEMO: return ECMULTIPLY_MEMO[k] else: result = ecmultiply(k // 2, multiply_by_2(P, p), p) ECMULTIPLY_MEMO[k] = result return result else: return add_points(P, ecmultiply((k - 1) // 2, multiply_by_2(P, p), p))
# Recursive function to multiply a point by a scalar def mulk(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: return mulk(k // 2, multiply_by_2(P, p), p) else: return add_points(P, mulk((k - 1) // 2, multiply_by_2(P, p), p))
# Generate a list of powers of two for faster access @lru_cache(maxsize=None) def generate_powers_of_two(hop_modulo): return [mpz(1 << pw) for pw in range(hop_modulo)]
t = time.ctime() sys.stdout.write("\033[01;33m") sys.stdout.write(f"[+] [Kangaroo]: {t}" + "\n") sys.stdout.flush()
# Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 2 ** (puzzle - 1) upper_range_limit = (2 ** puzzle) - 1 kangaroo_power = puzzle // 8 Nt = Nw = (2 ** kangaroo_power // puzzle) * puzzle + 8 DP_rarity = 8 * puzzle hop_modulo = (puzzle // 2) + 8
# Precompute powers of two for faster access powers_of_two = generate_powers_of_two(hop_modulo)
T, t, dt = [], [], [] W, w, dw = [], [], []
if len(compressed_public_key) == 66: X = mpz(compressed_public_key[2:66], 16) Y = x_to_y(X, mpz(compressed_public_key[:2]) - 2) else: print("[error] pubkey len(66/130) invalid!")
print(f"[+] [Puzzle]: {puzzle}") print(f"[+] [Lower range limit]: {lower_range_limit}") print(f"[+] [Upper range limit]: {upper_range_limit}") print("[+] [Xcoordinate]: %064x" % X) print("[+] [Ycoordinate]: %064x" % Y)
W0 = Point(X, Y) starttime = oldtime = time.time()
Hops = 0
# Worker function for point search def search_worker( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit ): global STOP_EVENT pid = os.getpid() core_number = pid % cpu_count() #Random seed Config #constant_prefix = b'' #back to no constant #constant_prefix = b'\xbc\x9b\x8cd\xfc\xa1?\xcf' #Puzzle 50 seed - 10-18s constant_prefix = b'\xbc\x9b\x8cd' prefix_length = len(constant_prefix) length = 8 ending_length = length - prefix_length ending_bytes = os.urandom(ending_length) random_bytes = constant_prefix + ending_bytes print(f"[+] [Core]: {core_number+1:02}, [Random seed]: {random_bytes}") random.seed(random_bytes) t = [ mpz( lower_range_limit + mpz(random.randint(0, upper_range_limit - lower_range_limit)) ) for _ in range(Nt) ] T = [mulk(ti) for ti in t] dt = [mpz(0) for _ in range(Nt)] w = [ mpz(random.randint(0, upper_range_limit - lower_range_limit)) for _ in range(Nt) ] W = [add_points(W0, mulk(wk)) for wk in w] dw = [mpz(0) for _ in range(Nw)]
Hops, Hops_old = 0, 0
oldtime = time.time() starttime = oldtime
while True: for k in range(Nt): Hops += 1 pw = T[k].x % hop_modulo dt[k] = powers_of_two[pw] solved = check(T[k], t[k], DP_rarity, T, t, W, w) if solved: STOP_EVENT.set() break t[k] = mpz(t[k]) + dt[k] # Use mpz here T[k] = add_points(POINTS_TABLE[pw], T[k])
for k in range(Nw): Hops += 1 pw = W[k].x % hop_modulo dw[k] = powers_of_two[pw] solved = check(W[k], w[k], DP_rarity, W, w, T, t) if solved: STOP_EVENT.set() break w[k] = mpz(w[k]) + dw[k] # Use mpz here W[k] = add_points(POINTS_TABLE[pw], W[k])
if STOP_EVENT.is_set(): break
# Main script if __name__ == "__main__": process_count = cpu_count() print(f"[+] [Using {process_count} CPU cores for parallel search]:")
# Create a pool of worker processes pool = Pool(process_count) results = pool.starmap( search_worker, [ ( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit, ) ] * process_count, ) pool.close() pool.join() Now it works. Thank you.
|
|
|
|
|
|
|
Advertised sites are not endorsed by the Bitcoin Forum. They may be unsafe, untrustworthy, or illegal in your jurisdiction.
|
nomachine
Member

Offline
Activity: 245
Merit: 12
|
 |
October 12, 2023, 10:01:26 PM Last edit: October 12, 2023, 10:52:29 PM by nomachine |
|
Yo @nomachine, what's that error dev/urandom thingy?
Computers are deterministic, predictable machines and are designed to blindly follow sets of instructions in a repeatable manner. This nature of computers has, of course, served us extremely well for most of the last century, but this design has a fundamental flaw: it cannot perform true random operations. True randomness is in quantum mechanics with time/space/gravity - I will stop here in order not to stray too far from the topic.... Most popular programming languages have some form of random number generator built in that programmers can use. These generators usually take as input the current date and time, encode this value using an algorithm and output a value so different from the input that we perceive them as random. A pseudorandom number generator, also known as a deterministic random bit generator, is an algorithm used to generate a sequence of numbers with properties that approximate those of truly random numbers. On all Unix-like systems, including all Linux distributions, there is a pseudo device file "/dev/urandom" If it doesn't exist, it might indicate a problem with system's configuration or user permissions. Or is not Linux. "Looks random to me" - is a pretty poor judgment for me in determining if something is random. 
|
|
|
|
albert0bsd
|
 |
October 13, 2023, 03:04:42 AM |
|
Yo @nomachine, what's that error dev/urandom thingy?
There is no problem with urandom it is enough random for all cryptographic purposes. Please all read the next article: https://www.2uo.de/myths-about-urandom/@nomachine one of the Hardware device that it is include most modern Intel processors, that is used to feed the entropy of the CSRNG of the linux kernel and windows core, use Thermodinamic properties to generate True random data: From: https://www.intel.com/content/www/us/en/developer/articles/guide/intel-digital-random-number-generator-drng-software-implementation-guide.htmlThe ES runs asynchronously on a self-timed circuit and uses thermal noise within the silicon to output a random stream of bits at the rate of 3 GHz. The ES needs no dedicated external power supply to run, instead using the same power supply as other core logic. The ES is designed to function properly over a wide range of operating conditions, exceeding the normal operating range of the processor. So that means that most of the modern PC are cable to generate True Random data.
|
|
|
|
nomachine
Member

Offline
Activity: 245
Merit: 12
|
 |
October 13, 2023, 04:51:59 AM Last edit: October 13, 2023, 09:04:46 AM by nomachine |
|
So that means that most of the modern PC are cable to generate True Random data.
I have been working on artificial intelligence research for years. If randomness is good enough to simulate reality and intelligence is part of reality(assuming that we ourselves are not living in a simulation), it should be good enough to simulate emotions and physical abilities - true intelligence. if you have a True randomness in your PC you will have possibility to have consciousness in your computer. To cut a long story short. If that is true (in absolute terms) , Singularity is close 
|
|
|
|
Ovixx
Newbie
Offline
Activity: 22
Merit: 0
|
 |
October 13, 2023, 07:19:58 AM Last edit: October 13, 2023, 09:19:45 AM by Ovixx |
|
Yo @nomachine, what's that error dev/urandom thingy?
................ If it doesn't exist, it might indicate a problem with system's configuration or user permissions. Or is not Linux. "Looks random to me" - is a pretty poor judgment for me in determining if something is random.  https://www.talkimg.com/images/2023/10/13/RExYZ.gif
|
|
|
|
nomachine
Member

Offline
Activity: 245
Merit: 12
|
 |
October 13, 2023, 07:44:36 AM Last edit: October 13, 2023, 08:05:02 AM by nomachine |
|
Yo @nomachine, what's that error dev/urandom thingy?
................ If it doesn't exist, it might indicate a problem with system's configuration or user permissions. Or is not Linux. "Looks random to me" - is a pretty poor judgment for me in determining if something is random.  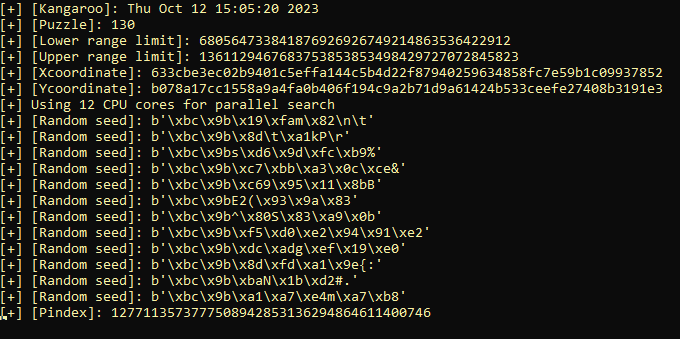 You can also setup smaller range in the frame in this mammoth # Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 680564733841876926926749214863536422911 #2 ** (puzzle - 1) upper_range_limit = 738823525229305890094942779208630272000 #(2 ** puzzle) - 1
or even smaller # Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 680564733841876926926749214863536422911 #2 ** (puzzle - 1) upper_range_limit = 680564733841876926926749314863536422911 #(2 ** puzzle) - 1
and so on...
|
|
|
|
|
frozenen
Newbie
Offline
Activity: 20
Merit: 0
|
 |
October 13, 2023, 10:37:39 AM |
|
@nomachine Your #130 lotto script works very easily, gonna leave it running on one of my pcs 24/7! I reckon: - [Lower range limit]: 1063382396627932698323045648224275660800
- [Upper range limit]: 1334544907768055536395422288521465954303
|
|
|
|
virus-cyber
Newbie
Offline
Activity: 22
Merit: 0
|
 |
October 13, 2023, 11:30:06 AM |
|
hi, can anyone install the KeyHunt-Cuda strid?
|
|
|
|
bestie1549
Jr. Member
Offline
Activity: 75
Merit: 5
|
 |
October 13, 2023, 12:43:58 PM |
|
that will not even be the case but the bounty alone is worth it if it would be paid in cryptocurrency but is the resources investment going to be covered with profits made afterwards? that is the real question here
|
|
|
|
Ovixx
Newbie
Offline
Activity: 22
Merit: 0
|
 |
October 13, 2023, 11:48:02 PM Last edit: October 14, 2023, 06:25:46 AM by Ovixx |
|
.......... You can also setup smaller range in the frame in this mammoth # Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 680564733841876926926749214863536422911 #2 ** (puzzle - 1) upper_range_limit = 738823525229305890094942779208630272000 #(2 ** puzzle) - 1
........... @nomachine ... the first time I tried to see if it works for me, now I changed the search range and the constant prefix of the seed. Thank's https://www.talkimg.com/images/2023/10/14/RkTR9.gif
|
|
|
|
digaran
Copper Member
Hero Member
   
Offline
Activity: 1330
Merit: 899
🖤😏
|
 |
October 14, 2023, 08:37:34 PM |
|
import gmpy2 as mpz from gmpy2 import powmod
# Define the ec_operations function def ec_operations(start_range, end_range, scalar_1, scalar_2, n, divide_1_by_odd=True, divide_1_by_even=True, divide_2_by_odd=True, divide_2_by_even=True): for i in range(start_range + (start_range%2), end_range, 2): # divide scalar 1 by odd or even numbers if i%2 == 0 and not divide_1_by_even: continue elif i%2 == 1 and not divide_1_by_odd: continue try: # calculate inverse modulo of i i_inv = powmod(i, n-2, n)
# multiply the scalar targets by i modulo n result_1 = scalar_2 * i_inv % n result_2 = scalar_1 * i_inv % n
# divide scalar 2 by odd or even numbers if i%2 == 0 and not divide_2_by_even: continue elif i%2 == 1 and not divide_2_by_odd: continue
# subtract the results sub_result = (result_2 - result_1) % n
# print results separately (f"{hex(result_1)[2:]}") (f"{hex(result_2)[2:]}") print(f"{i}-{hex(sub_result)[2:]}")
except ZeroDivisionError: pass
if __name__ == "__main__": # Set the targets and range for the operations scalar_1 = 0x000000000000000000000000000000000000000af55fc59c335c8ec67e66df97 scalar_2 = 0x000000000000000000000000000000000000000af55fc59c335c8ec67e66df8b
n = mpz.mpz("0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEBAAEDCE6AF48A03BBFD25E8CD0364141")
start_range = 2 end_range = 257
ec_operations(start_range, end_range, scalar_1, scalar_2, n) I always wondered when I work with target +1, 2, 3 etc what would be the results, so now I need to wonder no more. There are some interesting results when we start adding to our target and then divide. In the script above, if you start by scalar_1 +1 without changing scalar_2, running the script and only look at keys similar to n/2, n/4, n/6 etc, then keep adding to scalar_1 and run again to see where those n/? values appear. Note that when we don't know the actual 2 targets, we can always know the resulting keys, but when we change one target with a known key, then knowing the resulting keys would solve the key for us, that's what is needed, however there are ways and tricks to hit one previously unknown key in one of the new results if we keep changing our known key at scalar_1 or 2.
Something to work with. Let scalar_1 be : 0x000000000000000000000000000000000000000af55fc59c335c8ec67e66df8b Subtract this from it : af00000000000000000000000 Result: put at scalar_2 0x0000000000000000000000000000000000000000055fc59c335c8ec67e66df8b Now run and see the results, you will see af00000000000000000000000 being divided, so whatever we subtract from our target ( scalar_1 ), the results of script above will show us that number being divided even if we don't know it's key. Now since we can always know the resulting keys as long as the 2 targets are unknown and we already know the distance between them, but now I'm interested to find out more. Example: Subtracting this from puzzle 130, 0000000000000000000000000000000400000000000000000000000000000000 Result: (offset1) 0308360beeb0177961b04eccc33decdf63e23d205abc8ef6355d659d1313459ba7 Subtracting above from #130, result : (offset2) 0283aac9d18b994b94c0d267921573958682a061d033e89d2b0c4614c760755e60 Now what if we use offset1 and offset2 as our targets and do the divide and sub like the script above? If we could find a known key in the results, we can solve #130, or any private key. 😉
Chop chop, don't just stare and forget, also remember when you find the solution to solve any key, if you like your head to stay on your shoulders, hands off the people's coins. ey 😉
|
🖤😏
|
|
|
Ovixx
Newbie
Offline
Activity: 22
Merit: 0
|
 |
October 16, 2023, 08:04:42 AM |
|
@nomachine - for another puzzle, do I need to change anything in the script other than keyrange and pubkey? Why am I asking you? Because, in order to test, I put the public key from #64 and a very small keyrange, between f7051f26b09112d4 and f7051f2ab09112d4 (17799667353283269332:17799667370463138516) and it is obvious that the search is done outside the keyrange, in decimal, from 10000000000000000000 to 29999999999999999999 or even greater. https://www.talkimg.com/images/2023/10/16/RrO2l.gif
|
|
|
|
Baskentliia
Jr. Member
Offline
Activity: 32
Merit: 1
|
 |
October 16, 2023, 12:50:44 PM |
|
PUZZLE SOLVED: Wed Oct 11 12:13:21 2023, total time: 13.47 sec - WIF: -0000000000000000000000000000000000000000000000000022bd43c2e9354
nice catching again nomachine, is this programmable for 120th and up Yes. It takes about 3 minutes to start solving Puzzle 130 on my 12 Core - but will start. Here is code for Puzzle130 import sys import os import time import random import hashlib import gmpy2 from gmpy2 import mpz from functools import lru_cache import multiprocessing from multiprocessing import Pool, cpu_count
# Constants MODULO = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEFFFFFC2F) ORDER = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEBAAEDCE6AF48A03BBFD25E8CD0364141) GX = gmpy2.mpz(0x79BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F81798) GY = gmpy2.mpz(0x483ADA7726A3C4655DA4FBFC0E1108A8FD17B448A68554199C47D08FFB10D4B8)
# Define Point class class Point: def __init__(self, x=0, y=0): self.x = x self.y = y
PG = Point(GX, GY) ZERO_POINT = Point(0, 0)
# Function to multiply a point by 2 def multiply_by_2(P, p=MODULO): c = gmpy2.f_mod(3 * P.x * P.x * gmpy2.powmod(2 * P.y, -1, p), p) R = Point() R.x = gmpy2.f_mod(c * c - 2 * P.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to add two points def add_points(P, Q, p=MODULO): dx = Q.x - P.x dy = Q.y - P.y c = gmpy2.f_mod(dy * gmpy2.invert(dx, p), p) R = Point() R.x = gmpy2.f_mod(c * c - P.x - Q.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to calculate Y-coordinate from X-coordinate @lru_cache(maxsize=None) def x_to_y(X, y_parity, p=MODULO): Y = gmpy2.mpz(3) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
X = gmpy2.f_mod(tmp + 7, p)
Y = gmpy2.f_div(gmpy2.add(p, 1), 4) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
Y = tmp
if Y % 2 != y_parity: Y = gmpy2.f_mod(-Y, p)
return Y
# Function to compute a table of points def compute_point_table(): points = [PG] for k in range(255): points.append(multiply_by_2(points[k])) return points
POINTS_TABLE = compute_point_table()
# Global event to signal all processes to stop STOP_EVENT = multiprocessing.Event()
# Function to check and compare points for potential solutions def check(P, Pindex, DP_rarity, A, Ak, B, Bk): check = gmpy2.f_mod(P.x, DP_rarity) if check == 0: message = f"\r[+] [Pindex]: {mpz(Pindex)}" messages = [] messages.append(message) output = "\033[01;33m" + ''.join(messages) + "\r" sys.stdout.write(output) sys.stdout.flush() A.append(mpz(P.x)) Ak.append(mpz(Pindex)) return comparator(A, Ak, B, Bk) else: return False
# Function to compare two sets of points and find a common point def comparator(A, Ak, B, Bk): global STOP_EVENT result = set(A).intersection(set(B))
if result: sol_kt = A.index(next(iter(result))) sol_kw = B.index(next(iter(result))) difference = Ak[sol_kt] - Bk[sol_kw] HEX = "%064x" % difference t = time.ctime() pid = os.getpid() # Get the process ID core_number = pid % cpu_count() # Calculate the CPU core number total_time = time.time() - starttime print(f"\033[32m[+] PUZZLE SOLVED: {t}, total time: {total_time:.2f} sec, Core: {core_number+1:02} \033[0m") print(f"\033[32m[+] WIF: \033[32m {HEX} \033[0m") with open("KEYFOUNDKEYFOUND.txt", "a") as file: file.write("\n\nSOLVED " + t) file.write(f"\nTotal Time: {total_time:.2f} sec") file.write("\nPrivate Key (decimal): " + str(difference)) file.write("\nPrivate Key (hex): " + HEX) file.write( "\n-------------------------------------------------------------------------------------------------------------------------------------\n" )
STOP_EVENT.set() # Set the stop event to signal all processes
# Memoization for point multiplication ECMULTIPLY_MEMO = {}
# Function to multiply a point by a scalar def ecmultiply(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: if k in ECMULTIPLY_MEMO: return ECMULTIPLY_MEMO[k] else: result = ecmultiply(k // 2, multiply_by_2(P, p), p) ECMULTIPLY_MEMO[k] = result return result else: return add_points(P, ecmultiply((k - 1) // 2, multiply_by_2(P, p), p))
# Recursive function to multiply a point by a scalar def mulk(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: return mulk(k // 2, multiply_by_2(P, p), p) else: return add_points(P, mulk((k - 1) // 2, multiply_by_2(P, p), p))
# Generate a list of powers of two for faster access @lru_cache(maxsize=None) def generate_powers_of_two(hop_modulo): return [mpz(1 << pw) for pw in range(hop_modulo)]
# Worker function for point search def search_worker( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit ): global STOP_EVENT pid = os.getpid() core_number = pid % cpu_count() #Random seed Config #constant_prefix = b'' #back to no constant #constant_prefix = b'\xbc\x9b\x8cd\xfc\xa1?\xcf' #Puzzle 50 seed - 10-18s constant_prefix = b'\xbc\x9b' prefix_length = len(constant_prefix) length = 8 ending_length = length - prefix_length with open("/dev/urandom", "rb") as urandom_file: ending_bytes = urandom_file.read(ending_length) random_bytes = constant_prefix + ending_bytes print(f"[+] [Core]: {core_number+1:02}, [Random seed]: {random_bytes}") random.seed(random_bytes) t = [ mpz( lower_range_limit + mpz(random.randint(0, upper_range_limit - lower_range_limit)) ) for _ in range(Nt) ] T = [mulk(ti) for ti in t] dt = [mpz(0) for _ in range(Nt)] w = [ mpz(random.randint(0, upper_range_limit - lower_range_limit)) for _ in range(Nt) ] W = [add_points(W0, mulk(wk)) for wk in w] dw = [mpz(0) for _ in range(Nw)]
Hops, Hops_old = 0, 0
oldtime = time.time() starttime = oldtime
while True: for k in range(Nt): Hops += 1 pw = T[k].x % hop_modulo dt[k] = powers_of_two[pw] solved = check(T[k], t[k], DP_rarity, T, t, W, w) if solved: STOP_EVENT.set() break t[k] = mpz(t[k]) + dt[k] # Use mpz here T[k] = add_points(POINTS_TABLE[pw], T[k])
for k in range(Nw): Hops += 1 pw = W[k].x % hop_modulo dw[k] = powers_of_two[pw] solved = check(W[k], w[k], DP_rarity, W, w, T, t) if solved: STOP_EVENT.set() break w[k] = mpz(w[k]) + dw[k] # Use mpz here W[k] = add_points(POINTS_TABLE[pw], W[k])
if STOP_EVENT.is_set(): break
# Main script if __name__ == "__main__": os.system("clear") t = time.ctime() sys.stdout.write("\033[01;33m") sys.stdout.write(f"[+] [Kangaroo]: {t}" + "\n") sys.stdout.flush()
# Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 2 ** (puzzle - 1) upper_range_limit = (2 ** puzzle) - 1 kangaroo_power = puzzle // 8 Nt = Nw = (2 ** kangaroo_power // puzzle) * puzzle + 8 DP_rarity = 8 * puzzle hop_modulo = (puzzle // 2) + 8
# Precompute powers of two for faster access powers_of_two = generate_powers_of_two(hop_modulo)
T, t, dt = [], [], [] W, w, dw = [], [], []
if len(compressed_public_key) == 66: X = mpz(compressed_public_key[2:66], 16) Y = x_to_y(X, mpz(compressed_public_key[:2]) - 2) else: print("[error] pubkey len(66/130) invalid!")
print(f"[+] [Puzzle]: {puzzle}") print(f"[+] [Lower range limit]: {lower_range_limit}") print(f"[+] [Upper range limit]: {upper_range_limit}") print("[+] [Xcoordinate]: %064x" % X) print("[+] [Ycoordinate]: %064x" % Y)
W0 = Point(X, Y) starttime = oldtime = time.time()
Hops = 0
process_count = cpu_count() print(f"[+] [Using {process_count} CPU cores for parallel search]:")
# Create a pool of worker processes pool = Pool(process_count) results = pool.starmap( search_worker, [ ( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit, ) ] * process_count, ) pool.close() pool.join() I put only 2 bytes as a constant - the rest will be randomized through all CPU cores, since we don't know what the seed for 130 is. . . constant_prefix = b'\xbc\x9b' constant_prefix = b'' is all random ON the smaller puzzles it is easy to guess what the seed is. You need to restart and restart app and you will find out which is the fastest seed by repetition. The script will show exactly which seed and which core hit the WIF. You can experiment with different ones as you like. FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' I am getting error like this: C:\Users\abc\Desktop>py 4.py 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Using 8 CPU cores for parallel search]:
'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
multiprocessing.pool.RemoteTraceback: """ Traceback (most recent call last): File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 125, in worker result = (True, func(*args, **kwds)) ^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 51, in starmapstar return list(itertools.starmap(args[0], args[1])) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Users\abc\Desktop\4.py", line 219, in search_worker with open("/dev/urandom", "rb") as urandom_file: ^^^^^^^^^^^^^^^^^^^^^^^^^^ FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' """ The above exception was the direct cause of the following exception: Traceback (most recent call last): File "C:\Users\abc\Desktop\4.py", line 277, in <module> results = pool.starmap( ^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 375, in starmap return self._map_async(func, iterable, starmapstar, chunksize).get() ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 774, in get raise self._value FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' C:\Users\abc\Desktop> help me bro
|
|
|
|
unclevito
Jr. Member
Offline
Activity: 74
Merit: 4
|
 |
October 16, 2023, 01:31:09 PM |
|
PUZZLE SOLVED: Wed Oct 11 12:13:21 2023, total time: 13.47 sec - WIF: -0000000000000000000000000000000000000000000000000022bd43c2e9354
nice catching again nomachine, is this programmable for 120th and up Yes. It takes about 3 minutes to start solving Puzzle 130 on my 12 Core - but will start. Here is code for Puzzle130 import sys import os import time import random import hashlib import gmpy2 from gmpy2 import mpz from functools import lru_cache import multiprocessing from multiprocessing import Pool, cpu_count
# Constants MODULO = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEFFFFFC2F) ORDER = gmpy2.mpz(0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEBAAEDCE6AF48A03BBFD25E8CD0364141) GX = gmpy2.mpz(0x79BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F81798) GY = gmpy2.mpz(0x483ADA7726A3C4655DA4FBFC0E1108A8FD17B448A68554199C47D08FFB10D4B8)
# Define Point class class Point: def __init__(self, x=0, y=0): self.x = x self.y = y
PG = Point(GX, GY) ZERO_POINT = Point(0, 0)
# Function to multiply a point by 2 def multiply_by_2(P, p=MODULO): c = gmpy2.f_mod(3 * P.x * P.x * gmpy2.powmod(2 * P.y, -1, p), p) R = Point() R.x = gmpy2.f_mod(c * c - 2 * P.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to add two points def add_points(P, Q, p=MODULO): dx = Q.x - P.x dy = Q.y - P.y c = gmpy2.f_mod(dy * gmpy2.invert(dx, p), p) R = Point() R.x = gmpy2.f_mod(c * c - P.x - Q.x, p) R.y = gmpy2.f_mod(c * (P.x - R.x) - P.y, p) return R
# Function to calculate Y-coordinate from X-coordinate @lru_cache(maxsize=None) def x_to_y(X, y_parity, p=MODULO): Y = gmpy2.mpz(3) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
X = gmpy2.f_mod(tmp + 7, p)
Y = gmpy2.f_div(gmpy2.add(p, 1), 4) tmp = gmpy2.mpz(1)
while Y > 0: if Y % 2 == 1: tmp = gmpy2.f_mod(tmp * X, p) Y >>= 1 X = gmpy2.f_mod(X * X, p)
Y = tmp
if Y % 2 != y_parity: Y = gmpy2.f_mod(-Y, p)
return Y
# Function to compute a table of points def compute_point_table(): points = [PG] for k in range(255): points.append(multiply_by_2(points[k])) return points
POINTS_TABLE = compute_point_table()
# Global event to signal all processes to stop STOP_EVENT = multiprocessing.Event()
# Function to check and compare points for potential solutions def check(P, Pindex, DP_rarity, A, Ak, B, Bk): check = gmpy2.f_mod(P.x, DP_rarity) if check == 0: message = f"\r[+] [Pindex]: {mpz(Pindex)}" messages = [] messages.append(message) output = "\033[01;33m" + ''.join(messages) + "\r" sys.stdout.write(output) sys.stdout.flush() A.append(mpz(P.x)) Ak.append(mpz(Pindex)) return comparator(A, Ak, B, Bk) else: return False
# Function to compare two sets of points and find a common point def comparator(A, Ak, B, Bk): global STOP_EVENT result = set(A).intersection(set(B))
if result: sol_kt = A.index(next(iter(result))) sol_kw = B.index(next(iter(result))) difference = Ak[sol_kt] - Bk[sol_kw] HEX = "%064x" % difference t = time.ctime() pid = os.getpid() # Get the process ID core_number = pid % cpu_count() # Calculate the CPU core number total_time = time.time() - starttime print(f"\033[32m[+] PUZZLE SOLVED: {t}, total time: {total_time:.2f} sec, Core: {core_number+1:02} \033[0m") print(f"\033[32m[+] WIF: \033[32m {HEX} \033[0m") with open("KEYFOUNDKEYFOUND.txt", "a") as file: file.write("\n\nSOLVED " + t) file.write(f"\nTotal Time: {total_time:.2f} sec") file.write("\nPrivate Key (decimal): " + str(difference)) file.write("\nPrivate Key (hex): " + HEX) file.write( "\n-------------------------------------------------------------------------------------------------------------------------------------\n" )
STOP_EVENT.set() # Set the stop event to signal all processes
# Memoization for point multiplication ECMULTIPLY_MEMO = {}
# Function to multiply a point by a scalar def ecmultiply(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: if k in ECMULTIPLY_MEMO: return ECMULTIPLY_MEMO[k] else: result = ecmultiply(k // 2, multiply_by_2(P, p), p) ECMULTIPLY_MEMO[k] = result return result else: return add_points(P, ecmultiply((k - 1) // 2, multiply_by_2(P, p), p))
# Recursive function to multiply a point by a scalar def mulk(k, P=PG, p=MODULO): if k == 0: return ZERO_POINT elif k == 1: return P elif k % 2 == 0: return mulk(k // 2, multiply_by_2(P, p), p) else: return add_points(P, mulk((k - 1) // 2, multiply_by_2(P, p), p))
# Generate a list of powers of two for faster access @lru_cache(maxsize=None) def generate_powers_of_two(hop_modulo): return [mpz(1 << pw) for pw in range(hop_modulo)]
# Worker function for point search def search_worker( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit ): global STOP_EVENT pid = os.getpid() core_number = pid % cpu_count() #Random seed Config #constant_prefix = b'' #back to no constant #constant_prefix = b'\xbc\x9b\x8cd\xfc\xa1?\xcf' #Puzzle 50 seed - 10-18s constant_prefix = b'\xbc\x9b' prefix_length = len(constant_prefix) length = 8 ending_length = length - prefix_length with open("/dev/urandom", "rb") as urandom_file: ending_bytes = urandom_file.read(ending_length) random_bytes = constant_prefix + ending_bytes print(f"[+] [Core]: {core_number+1:02}, [Random seed]: {random_bytes}") random.seed(random_bytes) t = [ mpz( lower_range_limit + mpz(random.randint(0, upper_range_limit - lower_range_limit)) ) for _ in range(Nt) ] T = [mulk(ti) for ti in t] dt = [mpz(0) for _ in range(Nt)] w = [ mpz(random.randint(0, upper_range_limit - lower_range_limit)) for _ in range(Nt) ] W = [add_points(W0, mulk(wk)) for wk in w] dw = [mpz(0) for _ in range(Nw)]
Hops, Hops_old = 0, 0
oldtime = time.time() starttime = oldtime
while True: for k in range(Nt): Hops += 1 pw = T[k].x % hop_modulo dt[k] = powers_of_two[pw] solved = check(T[k], t[k], DP_rarity, T, t, W, w) if solved: STOP_EVENT.set() break t[k] = mpz(t[k]) + dt[k] # Use mpz here T[k] = add_points(POINTS_TABLE[pw], T[k])
for k in range(Nw): Hops += 1 pw = W[k].x % hop_modulo dw[k] = powers_of_two[pw] solved = check(W[k], w[k], DP_rarity, W, w, T, t) if solved: STOP_EVENT.set() break w[k] = mpz(w[k]) + dw[k] # Use mpz here W[k] = add_points(POINTS_TABLE[pw], W[k])
if STOP_EVENT.is_set(): break
# Main script if __name__ == "__main__": os.system("clear") t = time.ctime() sys.stdout.write("\033[01;33m") sys.stdout.write(f"[+] [Kangaroo]: {t}" + "\n") sys.stdout.flush()
# Configuration for the puzzle puzzle = 130 compressed_public_key = "03633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852" # Puzzle 130 lower_range_limit = 2 ** (puzzle - 1) upper_range_limit = (2 ** puzzle) - 1 kangaroo_power = puzzle // 8 Nt = Nw = (2 ** kangaroo_power // puzzle) * puzzle + 8 DP_rarity = 8 * puzzle hop_modulo = (puzzle // 2) + 8
# Precompute powers of two for faster access powers_of_two = generate_powers_of_two(hop_modulo)
T, t, dt = [], [], [] W, w, dw = [], [], []
if len(compressed_public_key) == 66: X = mpz(compressed_public_key[2:66], 16) Y = x_to_y(X, mpz(compressed_public_key[:2]) - 2) else: print("[error] pubkey len(66/130) invalid!")
print(f"[+] [Puzzle]: {puzzle}") print(f"[+] [Lower range limit]: {lower_range_limit}") print(f"[+] [Upper range limit]: {upper_range_limit}") print("[+] [Xcoordinate]: %064x" % X) print("[+] [Ycoordinate]: %064x" % Y)
W0 = Point(X, Y) starttime = oldtime = time.time()
Hops = 0
process_count = cpu_count() print(f"[+] [Using {process_count} CPU cores for parallel search]:")
# Create a pool of worker processes pool = Pool(process_count) results = pool.starmap( search_worker, [ ( Nt, Nw, puzzle, kangaroo_power, starttime, lower_range_limit, upper_range_limit, ) ] * process_count, ) pool.close() pool.join() I put only 2 bytes as a constant - the rest will be randomized through all CPU cores, since we don't know what the seed for 130 is. . . constant_prefix = b'\xbc\x9b' constant_prefix = b'' is all random ON the smaller puzzles it is easy to guess what the seed is. You need to restart and restart app and you will find out which is the fastest seed by repetition. The script will show exactly which seed and which core hit the WIF. You can experiment with different ones as you like. FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' I am getting error like this: C:\Users\abc\Desktop>py 4.py 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Using 8 CPU cores for parallel search]:
'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
multiprocessing.pool.RemoteTraceback: """ Traceback (most recent call last): File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 125, in worker result = (True, func(*args, **kwds)) ^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 51, in starmapstar return list(itertools.starmap(args[0], args[1])) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Users\abc\Desktop\4.py", line 219, in search_worker with open("/dev/urandom", "rb") as urandom_file: ^^^^^^^^^^^^^^^^^^^^^^^^^^ FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' """ The above exception was the direct cause of the following exception: Traceback (most recent call last): File "C:\Users\abc\Desktop\4.py", line 277, in <module> results = pool.starmap( ^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 375, in starmap return self._map_async(func, iterable, starmapstar, chunksize).get() ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 774, in get raise self._value FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' C:\Users\abc\Desktop> help me bro This is not my code but it looks like you are using windows and '/dev/urandom' is a linux function that is also available in Windows Subsystem Ubuntu so I think you are using a linux program.in windows. I think a few pages back the creator of this program posted a version that does not use '/dev/urandom' that should work in windows.
|
|
|
|
hari1987
Newbie
Offline
Activity: 21
Merit: 0
|
 |
October 16, 2023, 02:54:58 PM |
|
can anyone kindly provide an example of public key subtraction code in python ?
|
|
|
|
Ovixx
Newbie
Offline
Activity: 22
Merit: 0
|
 |
October 16, 2023, 03:34:41 PM |
|
it does not work under windows, if you do not use the WSL subsystem
|
|
|
|
digaran
Copper Member
Hero Member
   
Offline
Activity: 1330
Merit: 899
🖤😏
|
 |
October 16, 2023, 06:14:45 PM |
|
can anyone kindly provide an example of public key subtraction code in python ?
Try this one. import secp256k1 as ice
target_public_key = "032f3342152eff6aca5e7314db6d3301a28d6a90ddcfd189f96babadc2a053d392" target = ice.pub2upub(target_public_key) num = 1000 # number of times. subtract = 1 # amount to subtract each time.
# Define the new generator point coordinates new_generator = (new_x_coordinate, new_y_coordinate) # Replace with actual coordinates
sustract_pub_new = ice.scalar_multiplication(subtract, new_generator) res = ice.point_loop_subtraction(num, target, subtract_pub_new)
for t in range(num + 1): h = (res[t * 65:t * 65 + 65]).hex() hc = ice.to_cpub(h) data = open("data-base.txt", "a") data.write(str(hc) + "\n") data.close() This will subtract 1G 1000 times from target, meaning if target is 2000, it will give you 1999, 1998, 1997... etc.
|
🖤😏
|
|
|
nomachine
Member

Offline
Activity: 245
Merit: 12
|
 |
October 16, 2023, 06:38:28 PM Last edit: October 16, 2023, 07:46:59 PM by nomachine |
|
@nomachine - for another puzzle, do I need to change anything in the script other than keyrange and pubkey? Why am I asking you? Because, in order to test, I put the public key from #64 and a very small keyrange, between f7051f26b09112d4 and f7051f2ab09112d4 (17799667353283269332:17799667370463138516) and it is obvious that the search is done outside the keyrange, in decimal, from 10000000000000000000 to 29999999999999999999 or even greater.  Pindex is essentially an identifier for the points being processed and helps keep track of the progress of the search and identify points that could potentially solve the cryptographic puzzle. It's used to match points from different sets and determine if they share the same x-coordinate. Since these values are generated randomly, they may not always fall within the specified range, especially if the random number generation is not constrained. The script employs a kangaroo twins hopping strategy to search for solutions. The hopping strategy involves adding a certain value to the x-coordinate of a point and then computing the next point in the sequence. Depending on the hopping value, it is possible for the point to move outside the defined range. I am getting error like this: C:\Users\abc\Desktop>py 4.py 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Using 8 CPU cores for parallel search]:
'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. 'clear' is not recognized as an internal or external command, operable program or batch file. - [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Puzzle]: 130
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Lower range limit]: 680564733841876926926749214863536422912
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Kangaroo]: Mon Oct 16 15:48:30 2023
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
- [Puzzle]: 130
- [Lower range limit]: 680564733841876926926749214863536422912
- [Upper range limit]: 1361129467683753853853498429727072845823
- [Xcoordinate]: 633cbe3ec02b9401c5effa144c5b4d22f87940259634858fc7e59b1c09937852
- [Ycoordinate]: b078a17cc1558a9a4fa0b406f194c9a2b71d9a61424b533ceefe27408b3191e3
multiprocessing.pool.RemoteTraceback: """ Traceback (most recent call last): File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 125, in worker result = (True, func(*args, **kwds)) ^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 51, in starmapstar return list(itertools.starmap(args[0], args[1])) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Users\abc\Desktop\4.py", line 219, in search_worker with open("/dev/urandom", "rb") as urandom_file: ^^^^^^^^^^^^^^^^^^^^^^^^^^ FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' """ The above exception was the direct cause of the following exception: Traceback (most recent call last): File "C:\Users\abc\Desktop\4.py", line 277, in <module> results = pool.starmap( ^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 375, in starmap return self._map_async(func, iterable, starmapstar, chunksize).get() ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "C:\Program Files\Python311\Lib\multiprocessing\pool.py", line 774, in get raise self._value FileNotFoundError: [Errno 2] No such file or directory: '/dev/urandom' C:\Users\abc\Desktop> help me bro Go again there and copy/paste script - it is updated several times. The new version has a Core list for each seed. https://bitcointalk.org/index.php?topic=1306983.msg62978550#msg62978550Use "cls" instead of "clear" in Windows  p.s. If someone has time (i'm sold out right now) , it wouldn't be a bad idea to make the same program in C++ with the same seed capabilities and to work above Puzzle 125 . . . It would work incomparably faster. 
|
|
|
|
|
|